RTIC Kanban
City Map Board
1. Import header files
- #import <NavinfoKit/NavinfoKit.h>
2. Configure APIKEY
Refer to Project Configuration Instructions.
3. Define NavinfoTrafficGraphicSearch
Define the traffic kanban search object NavinfoTrafficGraphicSearch and inherit the search protocol <NavinfoTrafficSearchDelegate>.
4. Construct NavinfoTrafficGraphicSearch
Construct the traffic kanban search object NavinfoTrafficGraphicSearch and set the proxy.
- _tgSearch = [[NavinfoTrafficGraphicSearch alloc] init];
_tgSearch.delegate = self;
5. Set the city map kanban search query parameters
The request parameter class of the city map kanban query is NavinfoTrafficCityGraphicQuery, the cityName (city name) is a mandatory parameter, and the adcode/name is supported. The example is "Beijing" or "110100". Please refer to the API documentation for other parameters.
- NavinfoTrafficCityGraphicQuery *query = [NavinfoTrafficCityGraphicQuery new];
query.cityName = @"Dalian";
6. Initiate search query parameters
Initiate a search request by calling the cityGraphicSearch method of NavinfoTrafficGraphicSearch.
- [_tgSearch cityGraphicSearch:query];
7. Processing data in callbacks
Proxy method when the query is successful.
- - (void)onTrafficCityGraphicSearch:(NavinfoTrafficGraphicSearch * _Nonnull)graphicSearch
result:(NavinfoTrafficCityGraphicResult * _Nullable)result
error:(NSError * _Nullable)error;
The NavinfoTrafficCityGraphicResult is returned, and the query result can be obtained at this time.
Description:
Use the result.cityGraphicData image data to display the current city map kanban.
- - (void)onTrafficCityGraphicSearch:(NavinfoTrafficGraphicSearch *)graphicSearch result:(NavinfoTrafficCityGraphicResult *)result
error:(NSError *)error {
if (error == nil) {
[self performSelectorInBackground:@selector(downloadImageData:) withObject:result.cityGraphicData];
}
}
8. Processing failed queries
Proxy method when the retrieval fails.
- - (void)onTrafficCityGraphicSearch:(NavinfoTrafficGraphicSearch * _Nonnull)graphicSearch
result:(NavinfoTrafficCityGraphicResult * _Nullable)result
error:(NSError * _Nullable)error;
In Error will return, and the callback function will get the reason for the failure.
- - (void)onTrafficCityGraphicSearch:(NavinfoTrafficGraphicSearch *)graphicSearch result:(NavinfoTrafficCityGraphicResult *)result
error:(NSError *)error {
if (error) {
NSLog(@"----> error");
}
}
Run the program, the effect is as shown below:
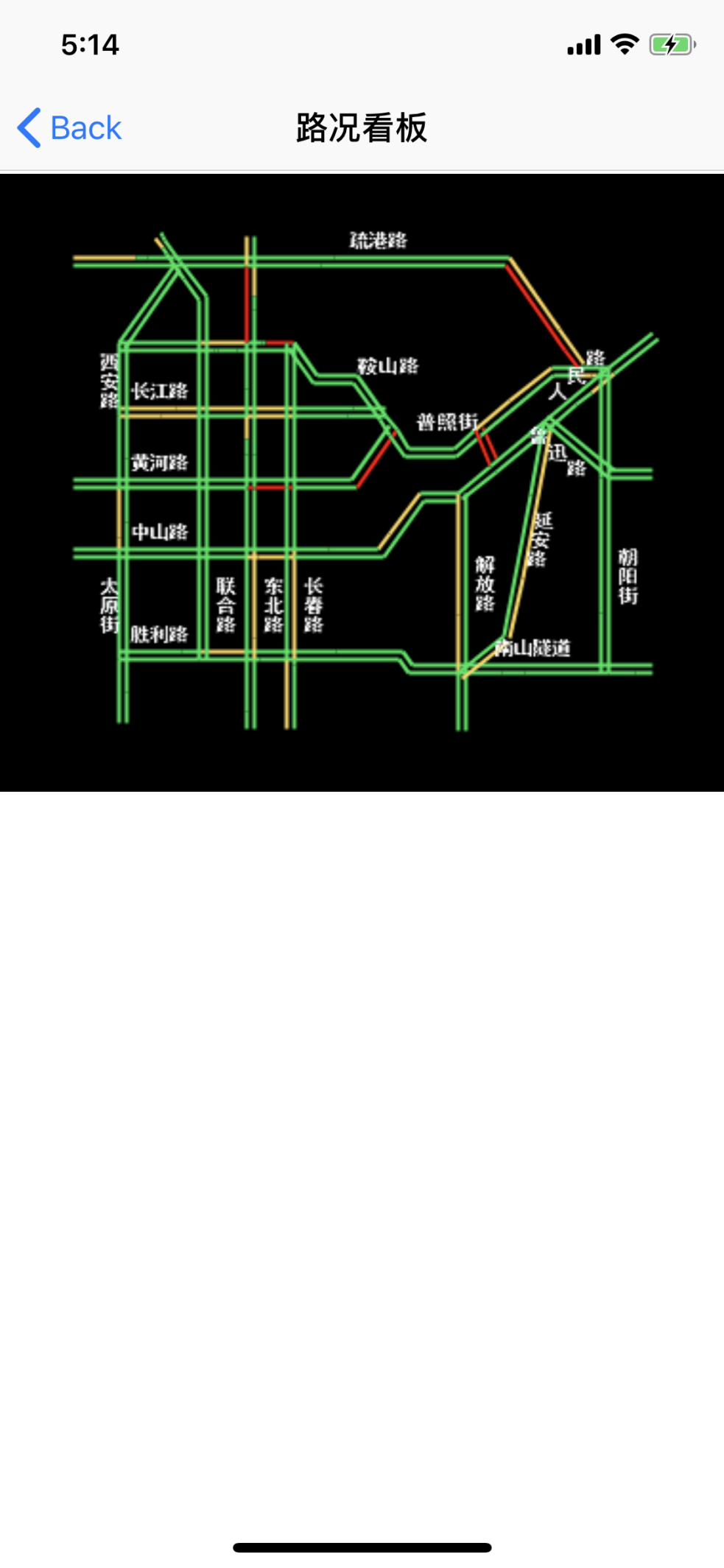
City List
1. Import header files
- #import <NavinfoKit/NavinfoKit.h>
2. Configure APIKEY
Refer to Project Configuration Instructions.
3. Define NavinfoTrafficGraphicSearch
Define the traffic kanban search object NavinfoTrafficGraphicSearch and inherit the search protocol <NavinfoTrafficSearchDelegate>.
4. Construct NavinfoTrafficGraphicSearch
Construct the traffic kanban search object NavinfoTrafficGraphicSearch and set the proxy.
- _tgSearch = [[NavinfoTrafficGraphicSearch alloc] init];
_tgSearch.delegate = self;
5. Set city list search query parameters
The request parameter class of the city map kanban query is NavinfoTrafficCityListQuery. This query request can be used directly without parameter setting.
- NavinfoTrafficCityListQuery *query = [NavinfoTrafficCityListQuery new];
6. Initiate search query parameters
Initiate a search request by calling the cityListSearch method of NavinfoTrafficGraphicSearch.
- [_tgSearch cityListSearch:query];
7. Processing data in callbacks
Proxy method when the query is successful.
- - (void)onTrafficCityListSearch:(NavinfoTrafficGraphicSearch * _Nonnull)graphicSearch
result:(NavinfoTrafficCityListResult * _Nullable)result
error:(NSError * _Nullable)error;
The NavinfoTrafficCityListResult is returned, and the query result can be obtained at this time.
Description:
Get a collection of city data via result.provinces.
- - (void)onTrafficCityGraphicSearch:(NavinfoTrafficGraphicSearch *)graphicSearch result:(NavinfoTrafficCityGraphicResult *)result
error:(NSError *)error {
if (error == nil) {
[self performSelectorInBackground:@selector(downloadImageData:) withObject:result.cityGraphicData];
}
}
8. Processing failed queries
Proxy method when the retrieval fails.
- - (void)onTrafficCityListSearch:(NavinfoTrafficGraphicSearch * _Nonnull)graphicSearch
result:(NavinfoTrafficCityListResult * _Nullable)result
error:(NSError * _Nullable)error;
In Error will return, and the callback function will get the reason for the failure.
- - (void)onTrafficCityListSearch:(NavinfoTrafficGraphicSearch *)graphicSearch result:(NavinfoTrafficCityListResult *)result error:(
NSError *)error {
if (error) {
NSLog(@"----> error");
}
}
The operation effect diagram is as follows:
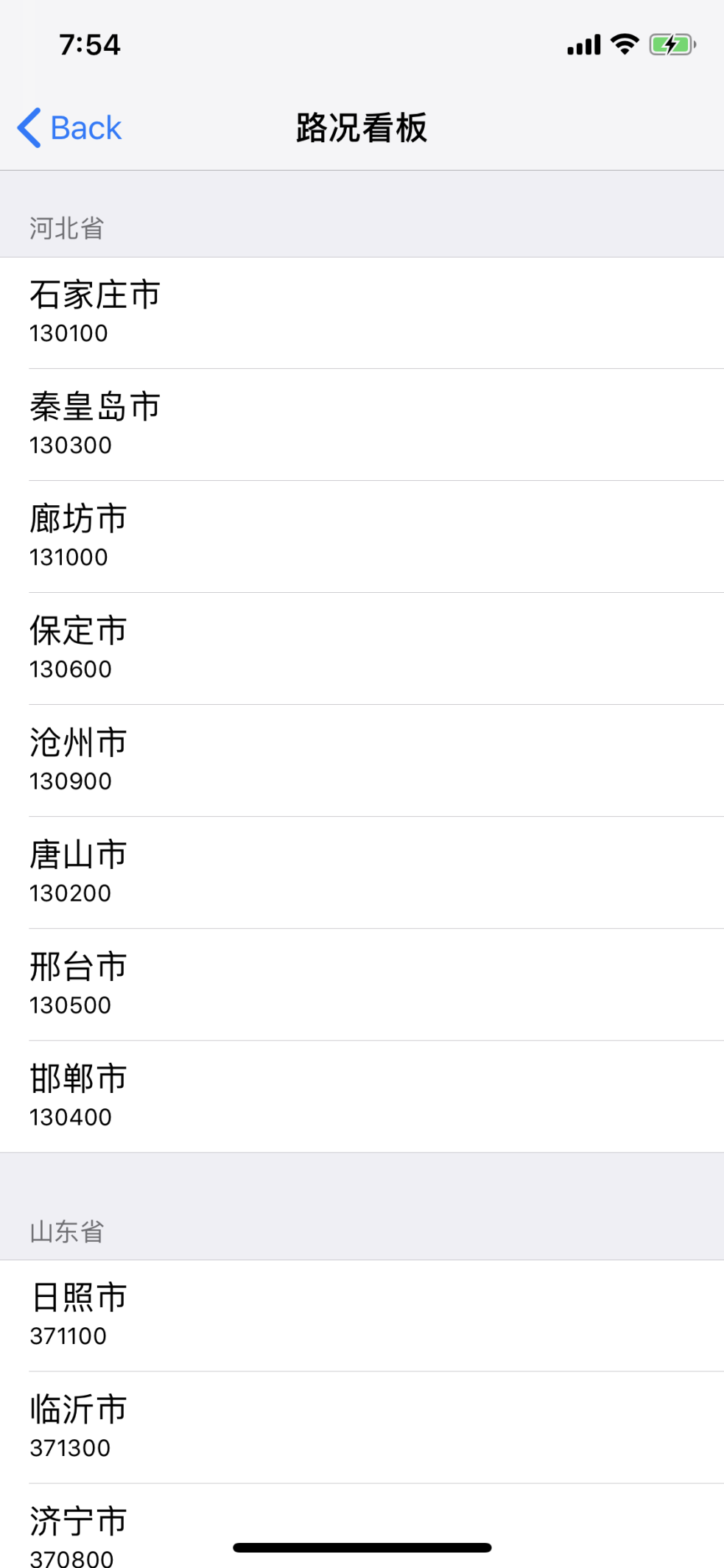