Development Guide
HUD Navigation Mode
HUD Navigation Mode Location:
Overview
NavInfo Map Navigation Client Application Development SDK [Navinfo Navigation SDK for iOS] is a map navigation client application development kit based on iOS 8.0 and above. Users can easily construct a variety of interactive applications based on map navigation. The four-dimensional map navigation client application product development SDK provides basic functions such as 3D map display and operation, location information search, navigation calculation, navigation process management, etc., to meet the various application needs of different users.
Feature introduction and experience
-
Simulation Navigation
(For complete code, see MBNaviController.m in the SDKDemo project) // real-time navigation If (naviMapView == nil) { // Map does not exist naviMapView=[[MBMapView alloc]initWithFrame:(0,120.0,kMainScreenSizeWidth, kMainScreenSizeHeight - 120.0 - 55.0)]; [self.view addSubview:naviMapView]; // Create a car logo layer MBModelOverlay *car = [[MBModelOverlay alloc] initWithFilePath:@"res/red_car.obj"]; carHead = MBNaviHeadNorthUp; } // Initialize the navigation module MBNaviSession *naviSession = [MBNaviSession sharedInstance]; naviSession.enableSound = YES; naviSession.delegate = self; // Callback function completed by route calculation - (void)naviSessionResult:(MBRouteCollection *)routes { // Calculate the road to complete // Get the routes collection, the routeBases in this collection, need to use when initiating navigation to obtain data for (MBOverlay *overlay in naviMapView.overlays) { [naviMapView removeOverlay:overlay]; } if (routes.routeBases.count > 0) { MBRouteOverlay *routeOverlay = nil; for (MBRouteBase *base in routes.routeBases) { routeOverlay = [[MBRouteOverlay alloc] initWithRoute:base.getRouteBase]; routeOverlay.clickEnable = YES; [naviMapView addOverlay:routeOverlay]; } } [naviSession startSimulation]; } - (void)naviSessionTracking:(MBNaviSessionData *)sData { NSLog (@"current car location: %d, %d", sData.carPos.x, sData.carPos.y); NSLog (@"current head orientation: %ld", (long) sData.carOri); NSLog (@"current recommended scale: %ld", (long) sData.suggestedMapScale); NSLog (@" Is there a next turn: %@, and %ld meters turn", sData.hasTurn?@"Yes": @"No", (long)sData.turnIconDistance); NSLog(@"current steering ID: %ld", (long)sData.turnIcon); NSLog (@"Road name after turn: %@", sData.nextRoadName); NSLog (@"current road name: %@", sData.roadName); NSLog(@"\n"); }
-
HUD Navigation Mode
- (void)updateHudViewWith:(MBNaviSessionData *)realtimeData { if (!realtimeData) { return; } MBNaviSessionData *aData = realtimeData; if (realtimeData.hasTurn) { _hudView.roadNameLabel.text = [NSString stringWithFormat:@"%@", aData.nextRoadName]; } if (!aData.drifting) { [_hudView.destanceProgressView setProgress: realtimeData.turnIconProgress/128.0 animated:YES]; }
NSInteger turnId = aData.turnIcon; MBGpsInfo *gpsInfo = [FakeGpsTracker sharedGpsTracker].currentGPSInfo;
if(!aData.drifting && turnId != 0 && gpsInfo.valid) { if(turnId == 1) { _hudView.turn_icon.hidden = YES; _hudView.turn_title.hidden = NO; _hudView.turn_icon_title.hidden = YES; } else { _hudView.turn_icon.image = [[MBResource sharedResoure] imageForName:[NSString stringWithFormat:@"turn_icons_hud/turn_hud_icons%ld.png", (long)turnId]]; _hudView.turn_icon.hidden = NO; _hudView.turn_title.hidden = YES; _hudView.turn_icon_title.hidden = YES; } } else { _hudView.turn_icon.hidden = YES; _hudView.turn_title.hidden = NO; _hudView.turn_icon_title.hidden = NO; } NSString*str = [MBUtils distanceTextWithLength:aData.turnIconDistance]; Str = [str stringByReplacingOccurrencesOfString:@"metre" withString:@"metre"]; if(aData.turnIconDistance >= 0) { NSMutableAttributedString *attrString = [[NSMutableAttributedString alloc] initWithString:[NSString stringWithFormat:@"%@, enter ",str]]; if (_hudView.layout == HUDViewLayoutHorizontal) { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont100]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont56]} range:NSMakeRange(attrString.length - 5, 5)]; } else { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont160]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont80]} range:NSMakeRange(attrString.length - 5, 5)]; } if ([attrString.string containsString:@" "]) { [attrString deleteCharactersInRange:NSMakeRange(attrString.length - 5, 1)]; } _hudView.destanceLabel.attributedText = attrString; } else { _hudView.destanceLabel.text = @""; } if(gpsInfo.valid) { NSMutableAttributedString *attrString = [[NSMutableAttributedString alloc] initWithString:[NSString stringWithFormat:@"%3.0f km/h",aData.speed*3.6]]; if (_hudView.layout == HUDViewLayoutHorizontal) { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont56]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont40]} range:NSMakeRange(attrString.length - 4, 4)]; } else { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont80]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont40]} range:NSMakeRange(attrString.length - 4, 4)]; } _hudView.speedLabel.attributedText = attrString; } else { _hudView.speedLabel.text = @"Signal weak"; } const MBRoadCamera *camera = aData.firstCamera; NSInteger routeDistance = aData.routeLength - aData.travelledDistance; NSString *distanceStr = nil; if (routeDistance >= 1000) { distanceStr = [NSString stringWithFormat:@"remaining %.0f km", round(routeDistance / 1000.0)]; } else { distanceStr = [NSString stringWithFormat:@"remaining %@", [MBUtils distanceTextWithLength:routeDistance]]; } NSString *timeStr = nil; NSInteger hours = aData.remainingTime / 3600; NSInteger minutes = aData.remainingTime / 60 - hours*60; if (minutes > 60) { timeStr = [NSString stringWithFormat:@"%ld hours%ld minutes", (long)hours, (long)minutes]; } else if (minutes == 60){ timeStr = [NSString stringWithFormat:@"%ldhours",(long)hours]; } else { timeStr = [NSString stringWithFormat:@"%ldminutes",(long)minutes]; } if (_hudView.layout == HUDViewLayoutHorizontal) { _hudView.all_destanceLabel.text = [NSString stringWithFormat:@"%@\n%@",distanceStr,timeStr]; } else { _hudView.all_destanceLabel.text = [NSString stringWithFormat:@"%@, %@",distanceStr,timeStr]; }
if(camera && (camera.type == MBCameraType_speed || camera.type == MBCameraType_radar || camera.type == MBCameraType_mobile)) { [_hudView.speed_icon setTitle:[NSString stringWithFormat:@"%lu",(unsigned long)camera.speedLimit] forState:0]; [_hudView.speedProgressView setProgress:(500 - camera.distanceFromCar)/500.0 animated:YES]; _hudView.speed_icon.hidden = NO; _hudView.compassLabel.hidden = YES; _hudView.speedProgressView.hidden = NO; } else { _hudView.speed_icon.hidden = YES; _hudView.speedProgressView.hidden = YES; _hudView.compassLabel.hidden = NO; _hudView.compassLabel.text = [self getCarOriStr:aData.carOri]; } }
- (NSString *)getCarOriStr:(NSUInteger)ori { NSUInteger n = ori/45; NSUInteger d = ori%45; NSArray *arr = @[@"east", @"East by North", @"northeast", @"North by East", @"north",@"North by West",@"North Northwest",@"northwest", @"west", @"West by South", @"southwest", @"South by West", @"south", @"South by East", @"southeast", @"South by East"]; // NSString *str = [NSString stringWithFormat:@"%@%@",[arr objectAtIndex:(2*n+(d?1:0))%16],d?[NSString stringWithFormat:@"%lu°", (long)(n%2?45-d:d)]:@""]; return [arr objectAtIndex:(2*n+(d?1:0))%16]; } -
Multiple Routing
(For complete code, see MBNavigationBaseController.m of the SDKDemo project) // route query plan @property (nonatomic, strong) MBRoutePlan *routePlan; // Initialize MBRoutePlan _routePlan = [[MBRoutePlan alloc]init]; // Simulation starting point MBPoiFavorite *startPoint = [[MBPoiFavorite alloc]init]; MBPoint startPos = {11639061, 4001898}; startPoint.pos = *(&startPos); // simulation end point MBPoiFavorite *endPoint = [[MBPoiFavorite alloc]init]; MBPoint endPos = {11639743,3990885}; endPoint.pos = *(&endPos); // Set the starting point [_routePlan setStartPoint:startPoint]; // set the end point [_routePlan setEndPoint:endPoint]; // Set the calculation rules [_routePlan setRule:MBRouteRule_recommended]; // Start counting [naviSession startRoute:_routePlan routeMethod:MBNaviSessionRouteMethod_multipleResult]; // MBNaviSessionDelegate -(void)naviSessionRouteStarted{ // Start counting } -(void)naviSessionResult:(MBRouteCollection *)routes{ // Calculate the road to complete // Get the routes collection, the routeBases in this collection, need to use when initiating navigation to obtain data } - (void)naviSessionRouteFailed:(MBTRouterError)errCode moreDetails:(NSString*)details{ // Calculating the road failed }
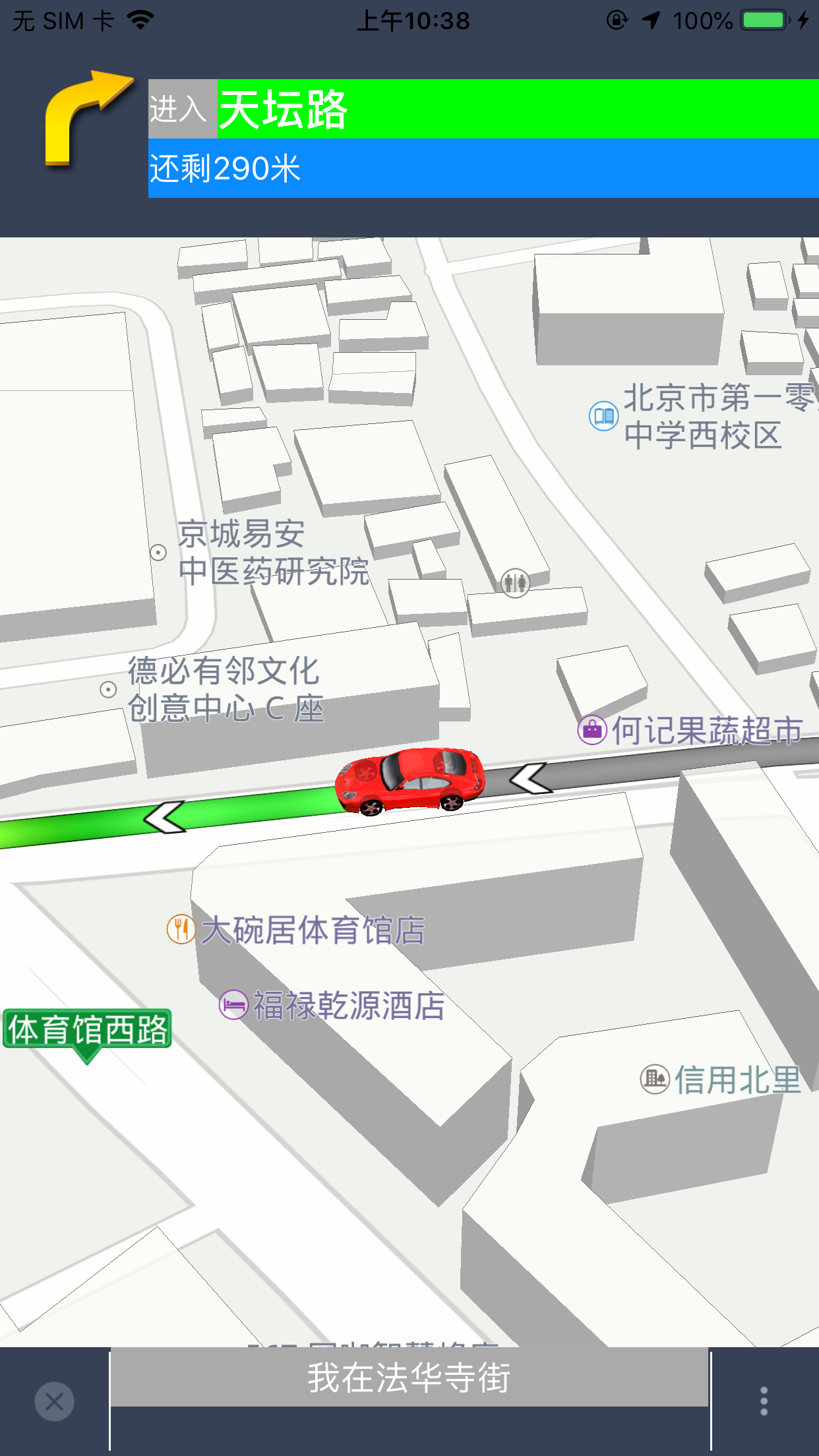