HUD Navigation Mode
HUD navigation is used for projection display during driving. This mode visually displays navigation information and is a safe and simple navigation mode. The navigation SDK provides you with a default HUD interface HUDView, as shown below:
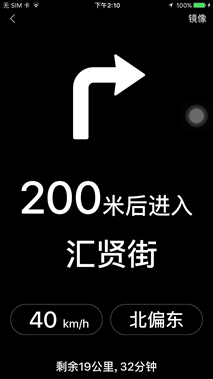
The following is an example of driving navigation, how to achieve HUD navigation.
Initialize
1. Initialize the HUDView object
- (void)initHudView { if (!self.hudView) { self.hudView = [[HUDView alloc] initWithFrame: self.bounds]; self.hudView.autoresizingMask = UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleHeight; self.hudView.layout = self.bounds.size.width > self.bounds.size.height ? HUDViewLayoutHorizontal : HUDViewLayoutVertical; [self addSubview: self.hudView]; } }
2. Update HUD based on navigation data
- (void)updateHudViewWith:(MBNaviSessionData *)realtimeData { if (!realtimeData) { return; } MBNaviSessionData *aData = realtimeData; if (realtimeData.hasTurn) { _hudView.roadNameLabel.text = [NSString stringWithFormat:@"%@", aData.nextRoadName]; } if (!aData.drifting) { [_hudView.destanceProgressView setProgress: realtimeData.turnIconProgress/128.0 animated:YES]; } NSInteger turnId = aData.turnIcon; MBGpsInfo *gpsInfo = [FakeGpsTracker sharedGpsTracker].currentGPSInfo; if(!aData.drifting && turnId != 0 && gpsInfo.valid) { if(turnId == 1) { _hudView.turn_icon.hidden = YES; _hudView.turn_title.hidden = NO; _hudView.turn_icon_title.hidden = YES; } else { _hudView.turn_icon.image = [[MBResource sharedResoure] imageForName:[NSString stringWithFormat:@"turn_icons_hud/turn_hud_icons%ld.png", (long)turnId]]; _hudView.turn_icon.hidden = NO; _hudView.turn_title.hidden = YES; _hudView.turn_icon_title.hidden = YES; } } else { _hudView.turn_icon.hidden = YES; _hudView.turn_title.hidden = NO; _hudView.turn_icon_title.hidden = NO;y } NSString*str = [MBUtils distanceTextWithLength:aData.turnIconDistance]; Str = [str stringByReplacingOccurrencesOfString:@"metre" withString:@"metre"]; if(aData.turnIconDistance >= 0) { NSMutableAttributedString *attrString = [[NSMutableAttributedString alloc] initWithString:[NSString stringWithFormat:@"%@, enter ",str]]; if (_hudView.layout == HUDViewLayoutHorizontal) { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont100]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont56]} range:NSMakeRange(attrString.length - 5, 5)]; } else { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont160]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont80]} range:NSMakeRange(attrString.length - 5, 5)]; } if ([attrString.string containsString:@" "]) { [attrString deleteCharactersInRange:NSMakeRange(attrString.length - 5, 1)]; } _hudView.destanceLabel.attributedText = attrString; } else { _hudView.destanceLabel.text = @""; } if(gpsInfo.valid) { NSMutableAttributedString *attrString = [[NSMutableAttributedString alloc] initWithString:[NSString stringWithFormat:@"%3.0f km/h",aData.speed*3.6]]; if (_hudView.layout == HUDViewLayoutHorizontal) { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont56]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont40]} range:NSMakeRange(attrString.length - 4, 4)]; } else { [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont80]} range:NSMakeRange(0, attrString.length)]; [attrString setAttributes:@{NSFontAttributeName: [UIFont mbMediumFont40]} range:NSMakeRange(attrString.length - 4, 4)]; } _hudView.speedLabel.attributedText = attrString; } else { _hudView.speedLabel.text = @"Signal weak"; } const MBRoadCamera *camera = aData.firstCamera; NSInteger routeDistance = aData.routeLength - aData.travelledDistance; NSString *distanceStr = nil; if (routeDistance >= 1000) { distanceStr = [NSString stringWithFormat:@"remaining %.0f km", round(routeDistance / 1000.0)]; } else { distanceStr = [NSString stringWithFormat:@"remaining %@", [MBUtils distanceTextWithLength:routeDistance]]; } NSString *timeStr = nil; NSInteger hours = aData.remainingTime / 3600; NSInteger minutes = aData.remainingTime / 60 - hours*60; if (minutes > 60) { timeStr = [NSString stringWithFormat:@"%ld hours%ld minutes", (long)hours, (long)minutes]; } else if (minutes == 60){ timeStr = [NSString stringWithFormat:@"%ldhours",(long)hours]; } else { timeStr = [NSString stringWithFormat:@"%ldminutes",(long)minutes]; } if (_hudView.layout == HUDViewLayoutHorizontal) { _hudView.all_destanceLabel.text = [NSString stringWithFormat:@"%@\n%@",distanceStr,timeStr]; } else { _hudView.all_destanceLabel.text = [NSString stringWithFormat:@"%@, %@",distanceStr,timeStr]; } if(camera && (camera.type == MBCameraType_speed || camera.type == MBCameraType_radar || camera.type == MBCameraType_mobile)) { [_hudView.speed_icon setTitle:[NSString stringWithFormat:@"%lu",(unsigned long)camera.speedLimit] forState:0]; [_hudView.speedProgressView setProgress:(500 - camera.distanceFromCar)/500.0 animated:YES]; _hudView.speed_icon.hidden = NO; _hudView.compassLabel.hidden = YES; _hudView.speedProgressView.hidden = NO; } else { _hudView.speed_icon.hidden = YES; _hudView.speedProgressView.hidden = YES; _hudView.compassLabel.hidden = NO; _hudView.compassLabel.text = [self getCarOriStr:aData.carOri]; } } - (NSString *)getCarOriStr:(NSUInteger)ori { NSUInteger n = ori/45; NSUInteger d = ori%45; NSArray *arr = @[@"east", @"East by North", @"northeast", @"North by East", @"north",@"North by West",@"North Northwest",@"northwest", @"west", @"West by South", @"southwest", @"South by West", @"south", @"South by East", @"southeast", @"South by East"]; // NSString *str = [NSString stringWithFormat:@"%@%@",[arr objectAtIndex:(2*n+(d?1:0))%16],d?[NSString stringWithFormat:@"%lu°", (long)(n%2?45-d:d)]:@""]; return [arr objectAtIndex:(2*n+(d?1:0))%16]; }
Load HUD View in the Driving Page
- (RouteNaviView *)naviView { return (RouteNaviView *)self.view; } - (void)viewDidLoad { [super viewDidLoad]; [RouteModel2 shared].naviSessionDelegate = self; [self.naviView showHudView]; }
Open Navigation
Enable navigation (real-time or simulation) in the callback function for successful route planning.
- (void)routeModelNaviSessionTracking:(MBNaviSessionData*)data { [super routeModelNaviSessionTracking:data]; if (self.naviView.hudView && self.naviView.hudView.superview) { [self.naviView updateHudViewWith:data]; } }