Location:
Overview
NavInfo Map Navigation Client Application Development SDK [Navinfo Navigation SDK for iOS] is a set based on iOS Map navigation client application product development kit for version 8.0 and above. Users can easily construct a variety of interactive applications based on map navigation. The four-dimensional map navigation client application product development SDK provides basic functions such as 3D map display and operation, location information search, navigation calculation, navigation process management, etc., to meet the various application needs of different users.
Feature introduction and experience
-
Map Display
(For complete code, see the MBMapController.m file of the SDKDemo project) #import <iNaviCore/MBMapView.h> @interface MBMapController () // map view @property (nonatomic ,strong) MBMapView *mapView; } - (void)viewDidLoad { [super viewDidLoad]; [self initMapView]; } - (void)initMapView { if (baseMapView == nil) { baseMapView = [[MBMapView alloc]initWithFrame:self.view.bounds]; [self.view addSubview:baseMapView]; } [baseMapView setZoomLevel:8.0 animated:YES]; baseMapView.delegate = self; }
-
Overlay
(For complete code, see MBNewSearchMapController.m of the SDKDemo project) // analog point MBPoint pos = {11617606,3971098}; // Offset of x and y axes CGPoint pivotPoint = {0.5,0.5}; // Initialize MBAnnotation annotation = [[MBAnnotation alloc] initWithZLevel:1 pos:pos iconId:8001 pivot:pivotPoint]; // Add MBAnnotation to MBMapView [mapView addAnnotation:annotation]; // Set the map center point to pos mapView.worldCenter = pos;
-
POI Search
(For complete code, see MBNewSearchListController.m of the SDKDemo project) // analog point MBPoint pos = {11637852,3986459}; // Initialize the MBPoiSearchSession class self.session = [MBPoiSearchSession defaultInstance]; // Set the query mode (default only online) self.session.preference = MBDataPreference_onlineOnly; self.session.isNearBy = YES; // Set the number of pages self.session.pageSize = 15; [self.session setCity:city]; // Set search keywords [self.session setKeyword:@"hotel"]; // Set the center point self.session.center = pos; // Start the query operation [self.session query]; [self.session setStartedBlock:^{ // Start the query }]; [self.session setEndedBlock:^{ // The query is successful, but the data is not loaded. }]; [self.session setCanceledBlock:^{ // cancel the query }]; [self.session setFailedBlock:^(MBPoiSearchError err, NSString* detail){ // Query failed }]; [self.session setLoadedBlock:^(NSArray *pois, NSArray *corrections, NSArray *cityDistributions, NSArray *citySuggestions, NSArray *districts) { // Query successful callback for (MBPoiItem *obj in pois){ NSLog(@"%@,%@",obj.name,obj.address); } NSLog(@"session pois:%@",pois); NSLog(@"correction:%@",corrections); NSLog(@"cityDistributions:%@",cityDistributions); NSLog(@"citySuggestions:%@",citySuggestions); NSLog(@"districts:%@",districts); }];
-
Offline Data
- (void)datastoreRefreshed:(MBDatastore *)ds { NSArray *dataStoreItems = ds.root.subnodes; NSMutableArray *provinceItems = [NSMutableArray arrayWithCapacity:dataStoreItems.count]; NSMutableArray *cityItems = [NSMutableArray array]; NSMutableArray *cityDataStoreItems = [NSMutableArray array]; for (MBDatastoreItem*provinceItem in dataStoreItems) { CTMLObj *provinceNode = [CTMLObj nodeWithOwnID:provinceItem.itemId name:provinceItem.name level:1]; [provinceItems addObject:provinceNode]; if (provinceItem.subnodes.count) { for (MBDatastoreItem* cityItem in provinceItem.subnodes) { MLNodelObj *cityNode = [MLNodelObj nodeWithParentID:provinceItem.itemId ownID:cityItem.itemId name:cityItem.name level:2 isleaf:YES isroot:NO isExpand:NO]; [cityItems addObject:cityNode]; [cityDataStoreItems addObject:cityItem]; } } else { [cityDataStoreItems addObject:provinceItems]; } provinceNode.subNodes = cityItems; } }
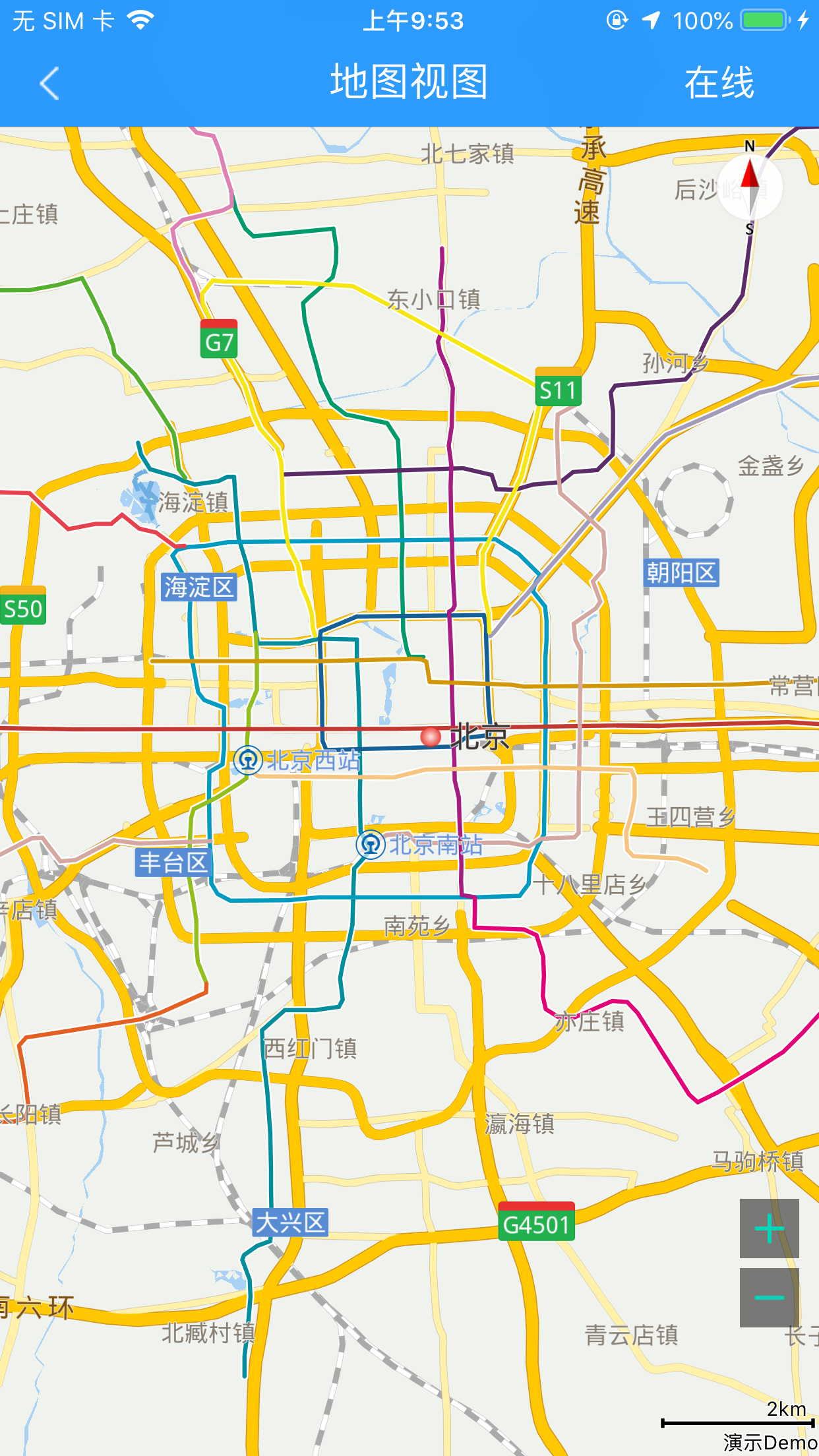