Draw lines and faces
Drawing Line
Why should you draw a line on the map? Because the identifiers that are marked on the map are connected to indicate that the identification points are related to the type of identification points. Implementation: Get a brush, get the relevant position identification points, and then draw a line between the relevant position identification points.
(For complete code, see MBOverlayerController.m of the SDKDemo project) // line // analog point MBPoint polyline1 = {11617606,3971098}; MBPoint polyline2 = {11660287,3971098}; MBPoint polylineP[2]; polylineP[0] = polyline1; polylineP[1] = polyline2; // Create MBPolylineOverlay MBPolylineOverlay *polylineLayer = [[MBPolylineOverlay alloc]initWithPoints:polylineP count:2 isClosed:NO]; // set the width [polylineLayer setWidth:20.0]; // set the line type [polylineLayer setStrokeStyle:MBStrokeStyle_11]; // Add MBPolylineOverlay to MBMapView [mapView addOverlay:polylineLayer];
Drawing line function display effect:
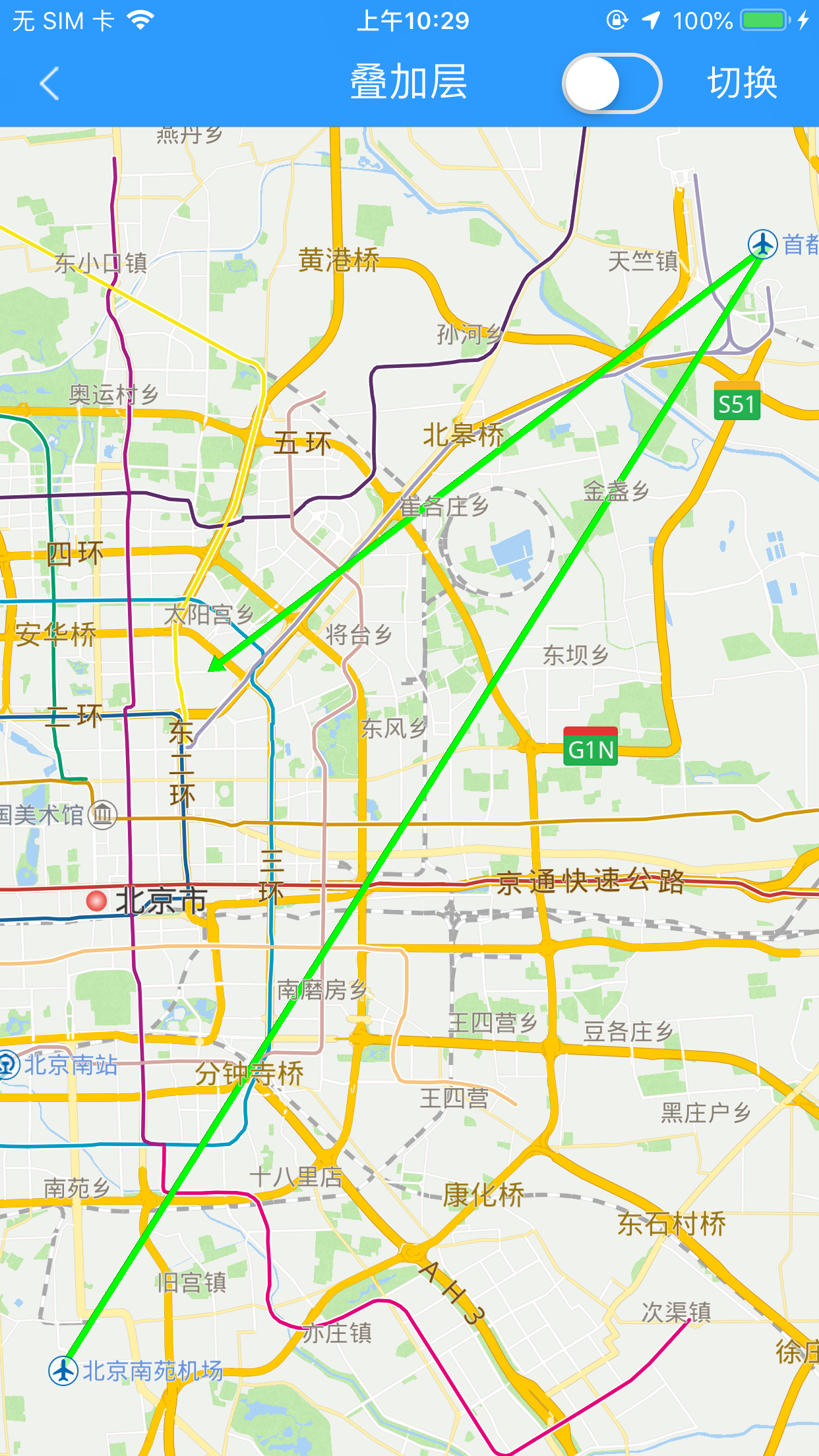
Drawing Circle
Why do you draw a circle on the map? Because the point to be marked on the map is highlighted, to highlight the point you need to identify. Implementation: Get a brush, get the relevant position identification point, and then draw a circle on the relevant position identification point.
(For complete code, see MBOverlayerController.m of the SDKDemo project) // round // analog point MBPoint center = {11617606,3971098}; // Create MBCircleOverlay and draw a solid circle with a radius of 1500 with the analog point as the center MBCircleOverlay *circleAreaOnly = [[MBCircleOverlay alloc]initWithCenter:center radius:1500.0]; // set style circleAreaOnly.style = MBCircleStyle_areaOnly; // Add MBCircleOverlay to MBMapView [mapView addOverlay:circleAreaOnly];
Draw a circle to show the effect:
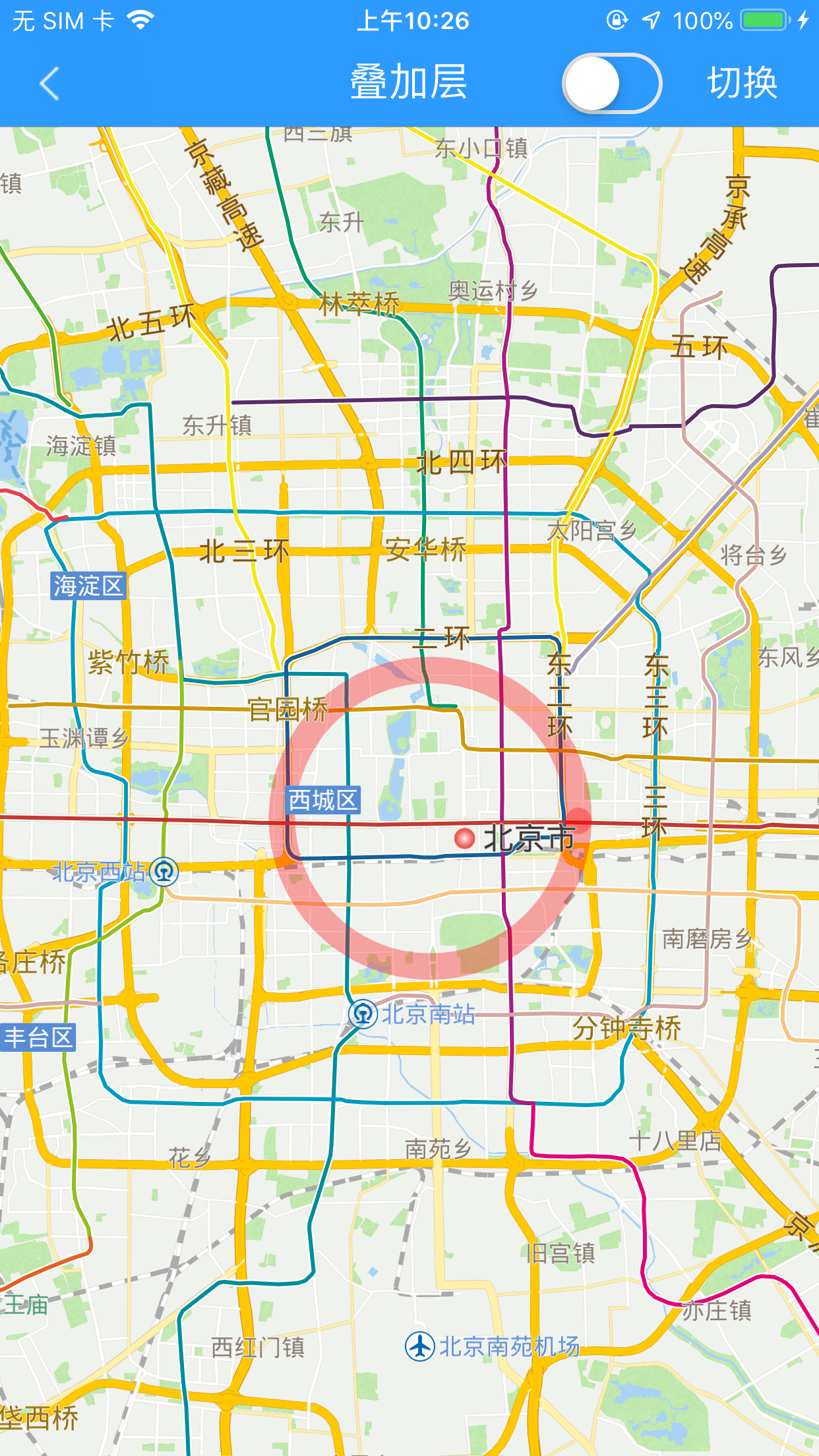
Draw Line
Why do you want to be on the map? Because it focuses on an area on the map to highlight areas that you need to be specifically identified. Implementation method: get a brush, get the relevant position identification point, then close the relevant position identification point, and color the area to draw the surface.
(For complete code, see MBOverlayerController.m of the SDKDemo project) // Simulate four points MBPoint point1 = {11637852,3986459}; MBPoint point2 = {11632209,3989693}; MBPoint point3 = {11631032,3999105}; MBPoint point4 = {11655760,3991267}; MBPoint polylineP[4]; polylineP[0] = point1; polylineP[1] = point2; polylineP[2] = point3; polylineP[3] = point4; // Create MBPolylineOverlay, pass in four points, draw the polyline from these four points MBPolylineOverlay *polylineLayer = [[MBPolylineOverlay alloc]initWithPoints:polylineP count:4 isClosed:NO]; // Set the line width [polylineLayer setWidth:20.0]; // set the line type [polylineLayer setStrokeStyle:strokeStyleIndex]; // Add MBPolylineOverlay to MBMapView [mapView addOverlay:polylineLayer];
Draw a line function to show the effect:
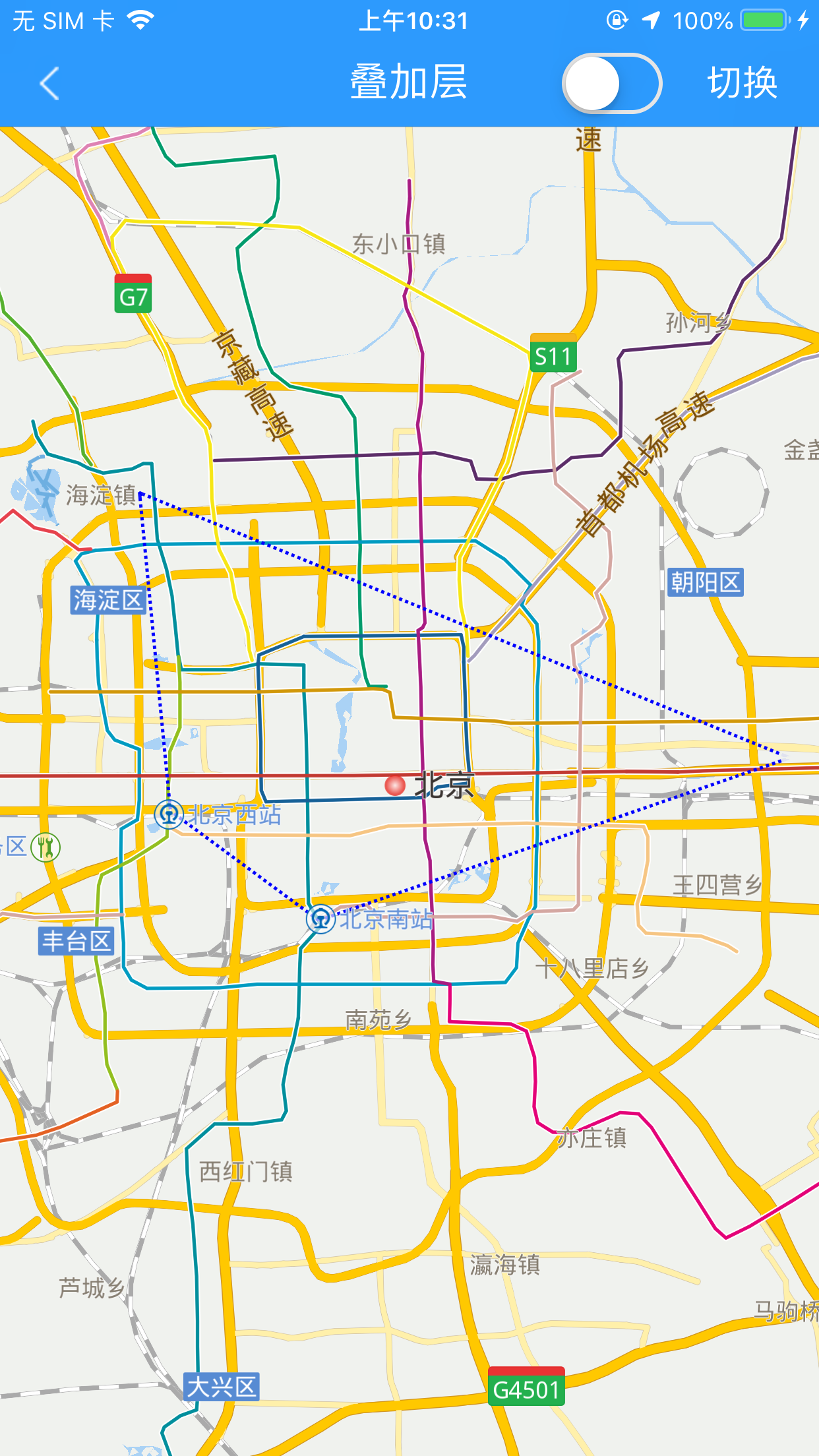
Draw Polygon
Why do you draw a polygon on the map? Because the markers that are marked on the map are connected and set to the corresponding color, it is used to indicate an area identified by these related points. Implementation method: Get a brush, get a set of position identification points of the relevant area, and then connect the relevant position identification points to form a closed area.
See the MBOverlayerController.m of the SDKDemo project for the complete code. // analog point MBPoint point1 = {11637852,3986459}; MBPoint point2 = {11632209,3989693}; MBPoint point3 = {11631032,3999105}; MBPoint point4 = {11655760,3991267}; MBPoint polygonP[4]; polygonP[0] = point1; polygonP[1] = point2; polygonP[2] = point3; polygonP[3] = point4; // Create MBPolygonOverlay, pass in four points, draw polygons from these four points MBPolygonOverlay *polygon = [[MBPolygonOverlay alloc]initWithPoints:polygonP count:4]; // Add MBPolygonOverlay to MBMapView [mapView addOverlay:polygon];
The effect of transparent polygons on the map:
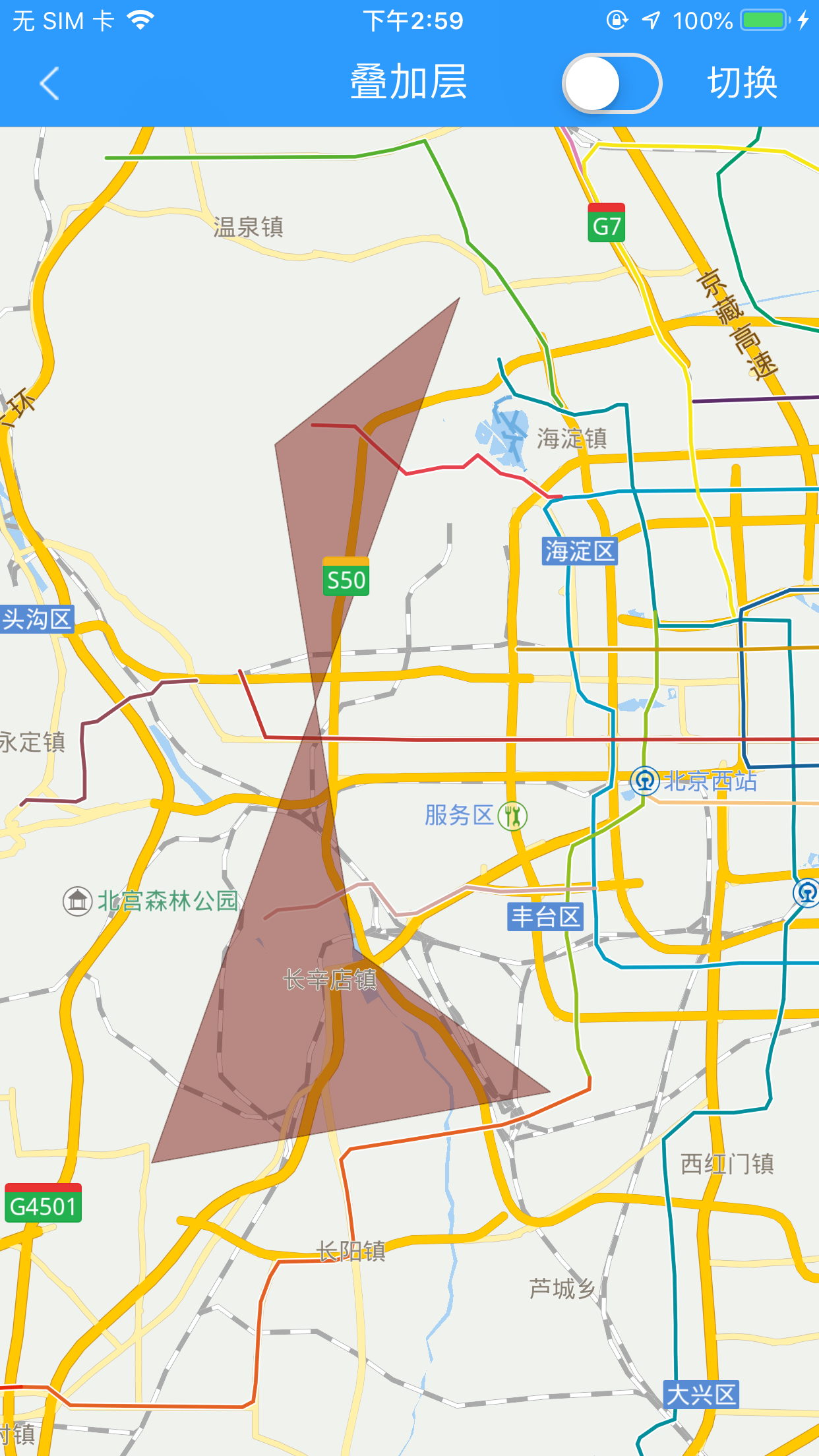