Overview
NavInfo Map Navigation Application Development SDK [Navinfo Navigation SDK for Android] is a map navigation client application product development kit based on Android 2.3 version and above. Users can easily construct a variety of interactive applications based on map navigation. The NavInfo map navigation application product development SDK provides basic functions such as 3D map display and operation, location information search, navigation calculation, navigation process management, etc. It can be combined with MPS (GPS, base station, WIFI hybrid positioning) interface to achieve map positioning and other functions, to meet Different application needs of different users.
Feature introduction and experience
-
Map Display
(For complete code, see the MapViewActivity.java file of the Demo project) // Custom map view private DemoMapView mDemoMapView; // Map rendering control class private MapRenderer mRenderer; /** * Show map, */ @Override protected void onCreate (Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.mapview); // Initialize the map initMap(); } /** * Initialize the map */ private void initMap () { try { // load the map mDemoMapView = (DemoMapView) findViewById(R.id.glView_mapview); mDemoMapView.setHandler(handler); } catch (Exception e) { e.printStackTrace(); new MessageBox(this, false).showDialog(e.getMessage()); } } /* * Custom map view, all map-related operations can be customized */ public class DemoMapView extends MapView { // Initialize the view used to enlarge the drawing // Start simulated navigation // End the simulation navigation // draw a magnified view // Draw the arrow specifying Maneuver // draw the camera // draw multiple routes // draw a single route // Set the route to open Tmc mode // show the route on the map // set the destination // Start counting // Control whether to lock the car // Get the current location of the car // Set the angle of the current car, used to update the angle of the car when navigating // Display a POI message at the specified location on the map // hide the specified route // delete all routes // Map zoom operation // Map zoom out // …… }
-
Overlay
(For complete code, see OverlayActivity.java in the Demo project) // Dot Point point = new Point(11638780, 3998076); // Create a point object CircleOverlay circle = new CircleOverlay(point, 0f); // Create a point centered on the point object mRenderer.addOverlay(circle);//Draw points on the map // bubble Vector2D pivot = new Vector2D(0.5f, 0.0f); // Set the offset of the bubble at the point Annotation an = new Annotation(1, point, 1101, pivot); // Create a bubble with an offset of pivot at the point CalloutStyle calloutStyle = an.getCalloutStyle(); // Get the bubble style calloutStyle.leftIcon = 0; // The icon to the left of the bubble is empty calloutStyle.rightIcon = 0; // The icon to the right of the bubble is empty an.setCalloutStyle(calloutStyle); // Set the display style for the bubble an.setTitle("custom POI point"); // display the title content for the bubble setting mRenderer.addAnnotation(an); // Add bubbles to the map an.showCallout(true); // Set the bubble to display
-
POI Search
(For complete code, see NewSearchActivity.java in the Demo project) /** * Initialization of the engine to be used here mainly uses the POI search engine and graphics engine */ private void init () { …… // Initialize the POI search engine mPoiSearch = new PoiSearch(); // Set the search mode: // onlineOnly online search offlineOnly offline search mPoiSearch.setDataPreference(DataPreference.onlineOnly); // Set the search city id mPoiSearch.setCity(cityName); // Set the search center point mPoiSearch.setCenter(mCenter); // Register search listener callback method // Ride on the map private void drawAnnotation() { mAnnotations.clear(); Vector2DF vec = new Vector2DF(0.9f, 0.5f); Point point = new Point(); int zlevel = 100; Bitmap icon = BitmapFactory.decodeResource(getResources(), R.drawable.gas); for (int i = 0; i < mPoiItems.size(); i++) { point.x = mPoiItems.get(i).position.x; point.y = mPoiItems.get(i).position.y; CustomAnnotation annot = new CustomAnnotation(zlevel, point, i, vec, icon); annot.setTitle(mPoiItems.get(i).name); mAnnotations.add(annot); } for (int i = 0; i < mAnnotations.size(); i++) { mDemoMapView.getMapRenderer().addAnnotation(mAnnotations.get(i)); } if (mPoiItems.size() > 0) { // Mobile Map Center mDemoMapView.setWorldCenter(mPoiItems.get(0).position); } } // Delete the point on the map private void clearAnnotation() { for (int i = 0; i < mAnnotations.size(); i++) { mDemoMapView.getMapRenderer().removeAnnotation(mAnnotations.get(i)); } }
-
Offline Data
// Initialize the data, add data load callback OfflineDataStoreManager.getInstance().initData(this); // data loading callback @Override public void onDataSuccess(DatastoreItem[] datastoreItems) { // Data loaded successfully progressBar.setVisibility(View.GONE); this.datastoreItems = datastoreItems; if (datastoreItems != null) { // Set the listView data source DownloadAdapter downloadAdapter = new DownloadAdapter(this, datastoreItems); mListView.setAdapter(downloadAdapter); } } @Override public void onDataFailure() { // Data loading failed progressBar.setVisibility(View.GONE); Toast.makeText(this, "refresh faile", Toast.LENGTH_SHORT).show(); } @Override public void onDataCancle() { // data loading canceled progressBar.setVisibility(View.GONE); Toast.makeText(this, "refresh cancel", Toast.LENGTH_SHORT).show(); }
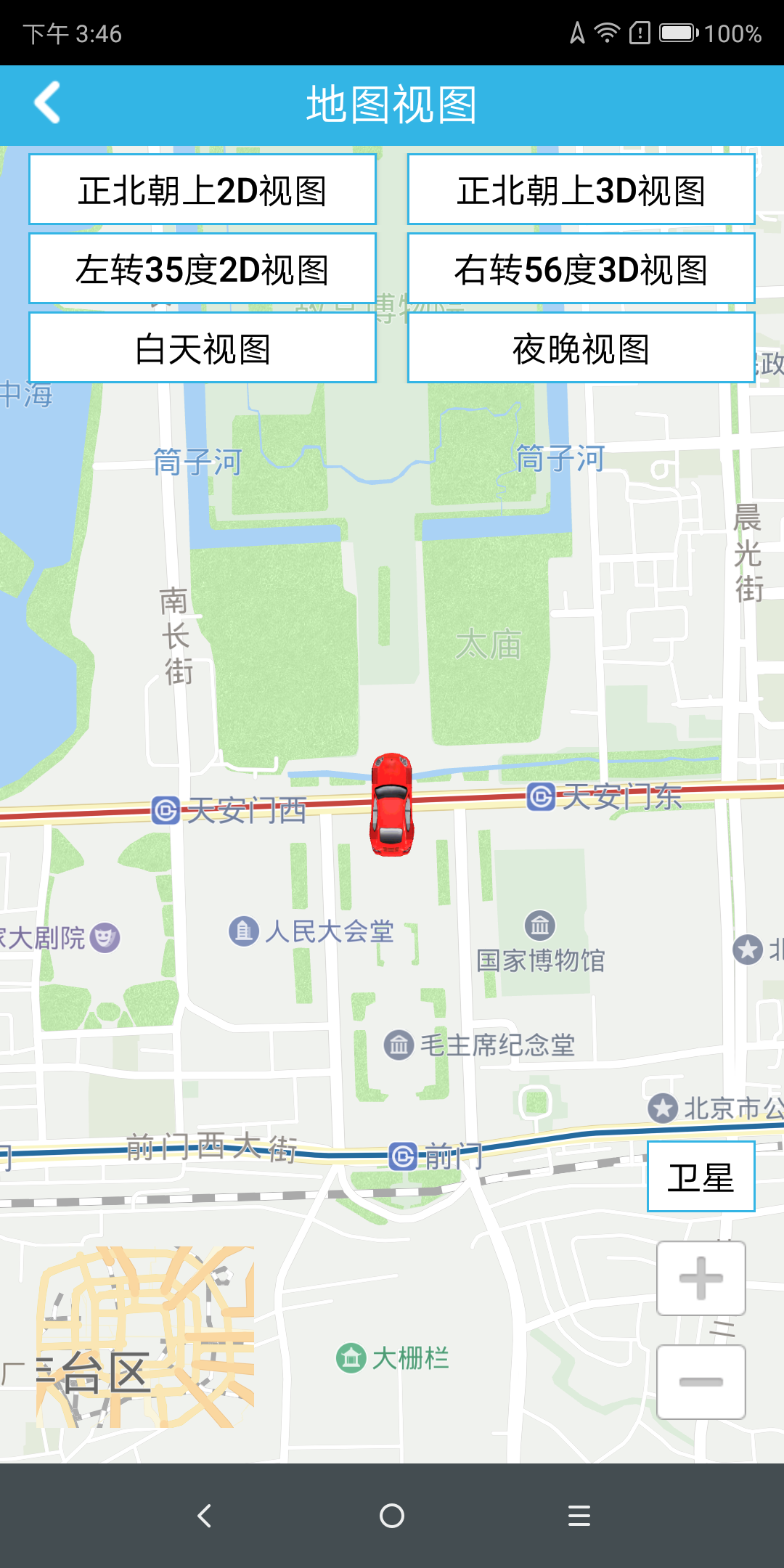