Location:
Overview
The NavInfo Map Java SDK is a set of application interfaces written in the Java language to support server-side map service development. Developers can call the SDK interface to easily access the services and data of NavInfo maps and quickly build feature-rich, interactive map-like application services. The SDK provides basic functions such as tram travel, path planning, batch calculation, and road events to meet the needs of different users.
The NavInfo Map Java SDK is compatible with JDK 1.7 and above.
Feature introduction and experience
-
Suggest
POISuggest mPOISuggest=new POISuggest(); // Set the request parameters, mPOISuggest.setQuery(POISuggest.Query.newQuery("Food", "Beijing")); // Set the request to return the result listener interface mPOISuggest.setListener(new POISuggest.Listener() { @Override public void onSuccess(POISuggestResult result) { //Thread is an asynchronous thread //The result returns successfully } @Override public void onFail(final APIStatus status) { //Result failed } }); // Start search suggestions mPOISuggest.search();
-
Charging Station Search
EVSearch mEvSearch = new EVSearch(); // Setting parameters mEvSearch.setQuery(EVSearch.newQuery("Tesla"))); mEvSearch.setListener(new EVSearch.Listener() { @Override public void onSuccess(final POISearchResult result) { // Search succeeded } @Override public void onFail(APIStatus status) { // search failed } }); // Initiate a search mEvSearch.search();
-
Vehicle Range
// Set parameters and results listener, initiate a search EVRange.searchRange(EVRange.Query.newQuery(new GeoPoint(116.39750, 39.90850), 8), new EVRange.Listener() { @Override public void onSuccess(final GeoPolygon range) { // Get the scope is successful } @Override public void onFail(final APIStatus status) { // Get the scope failed } });
-
Routing
// Set the parameters starting point and end point coordinates array GeoPoint[] geoPointStarts=new GeoPoint[]{new GeoPoint(116.34,40.45),new GeoPoint(116.35,40.54)}; GeoPoint[] geoPointEnds=new GeoPoint[]{new GeoPoint(116.45,40.34),new GeoPoint(116.46,40.35)}; // Initiate a request BatchRoutePlan.searchBatchRoute(BatchRoutePlan.newQuery(geoPointStarts, geoPointEnds), new BatchRoutePlan.Listener() { @Override public void onSuccess(final BatchRouteResult result) { // request succeeded } @Override public void onFail(final APIStatus status) { // Request failed } });
-
Traffic Kanban
//Get traffic kanban based on city name. For example, “Beijing” or “Beijing” GraphICSearch.searchGraphIC(GraphICSearch.Query.newQuery("BEIJING"), new GraphICSearch.GraphICListener() { @Override public void onSuccess(final byte[] result) { // Request success } @Override public void onFail(final APIStatus status) { //Request failed } });
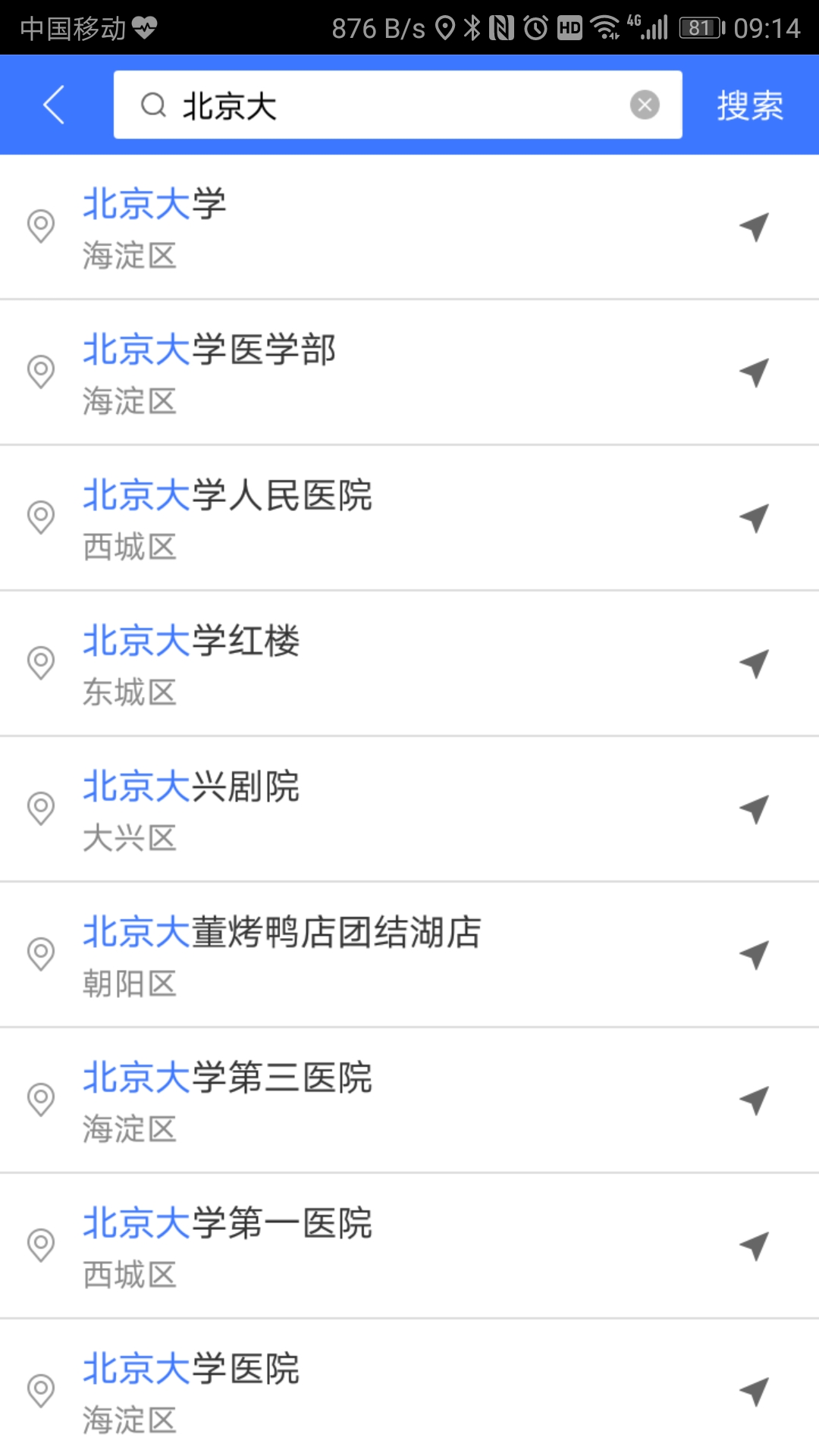