Search Service
[Navinfo Navigation SDK for Android] provides users with a total of 10 million levels of POI (Point of Interest) search service, search service online and offline search function. At present, the provided POI search methods include: keyword search, peripheral search, type search, search along the road, and the like.
1. The keyword search method is PoiSearch.searchWithKeyword;
2. The surrounding search method is PoiSearch.forceNearBy(true), set the forced use of the surrounding search, and then complete the search by calling PoiSearch.searchWithKeyword;
3. The search method along the way is PoiSearch.searchAlongRoute. The search engine needs to be initialized before using the search method. The initialization content includes: the size of the memory buffer used by the keyword search, the number of returned POIs, the coverage of the surrounding search, etc.;
4. Searching along the route requires navigation module support.
(For complete code, see NewSearchActivity.java in the Demo project) /** * Initialization of the engine to be used here mainly uses the POI search engine and graphics engine */ private void init () { …… // Initialize the POI search engine mPoiSearch = new PoiSearch(); // Set the search mode: // onlineOnly online search offlineOnly offline search mPoiSearch.setDataPreference(DataPreference.onlineOnly); // Set the search city id mPoiSearch.setCity(cityName); // Set the search center point mPoiSearch.setCenter(mCenter); // Register search listener callback method mPoiSearch.setListener(new PoiSearch.Listener() { @Override public void onPoiSearch(int event) { Log.d("search", event + ""); switch (event) { case PoiSearch.Event.queryFinished: Logger.i("luke finished event revieved"); break; case PoiSearch.Event.pageLoaded: try { // bubbles before the situation clearAnnotation(); mPoiItems.clear(); // For example, if you searched for 3 pages, poisearch contains all the information from 1 to 3 pages, so you need to calculate the position of the third page to get it. boolean flag1 = mPoiSearch.getTotalPoiItemSum() <= (mPageIndex + 1) * mPoiSearch.getPageSize(); int maxIndex = flag1 ? mPoiSearch.getTotalPoiItemSum() : (mPageIndex + 1) * mPoiSearch.getPageSize(); // Get the current number of load pages int index = mPoiSearch.getCurrentPoiNum(); if (index > 0) { int poiNumbers = mPoiSearch.getItemNum(BaseItem.ItemType.poi); if (poiNumbers > 0) { if (poiNumbers == 1) { PoiItem poiItem = (PoiItem) mPoiSearch.getItemByIndex(BaseItem.ItemType.poi, 0); // If it is an administrative area, jump to the administrative district. if (poiItem.type == PoiItem.ItemType.region) { RegionPoiItem regionPoiItem = (RegionPoiItem) poiItem; mDemoMapView.setCarPosition(regionPoiItem.center); mPoiSearch.setCity(regionPoiItem.completeName); mPoiSearch.setCenter(regionPoiItem.center); return; } } } } } …… } } // Ride on the map private void drawAnnotation() { mAnnotations.clear(); Vector2DF vec = new Vector2DF(0.9f, 0.5f); Point point = new Point(); int zlevel = 100; Bitmap icon = BitmapFactory.decodeResource(getResources(), R.drawable.gas); for (int i = 0; i < mPoiItems.size(); i++) { point.x = mPoiItems.get(i).position.x; point.y = mPoiItems.get(i).position.y; CustomAnnotation annot = new CustomAnnotation(zlevel, point, i, vec, icon); annot.setTitle(mPoiItems.get(i).name); mAnnotations.add(annot); } for (int i = 0; i < mAnnotations.size(); i++) { mDemoMapView.getMapRenderer().addAnnotation(mAnnotations.get(i)); } if (mPoiItems.size() > 0) { // Mobile Map Center mDemoMapView.setWorldCenter(mPoiItems.get(0).position); } } // delete the point on the map private void clearAnnotation() { for (int i = 0; i < mAnnotations.size(); i++) { mDemoMapView.getMapRenderer().removeAnnotation(mAnnotations.get(i)); } } }
Search interface after running:
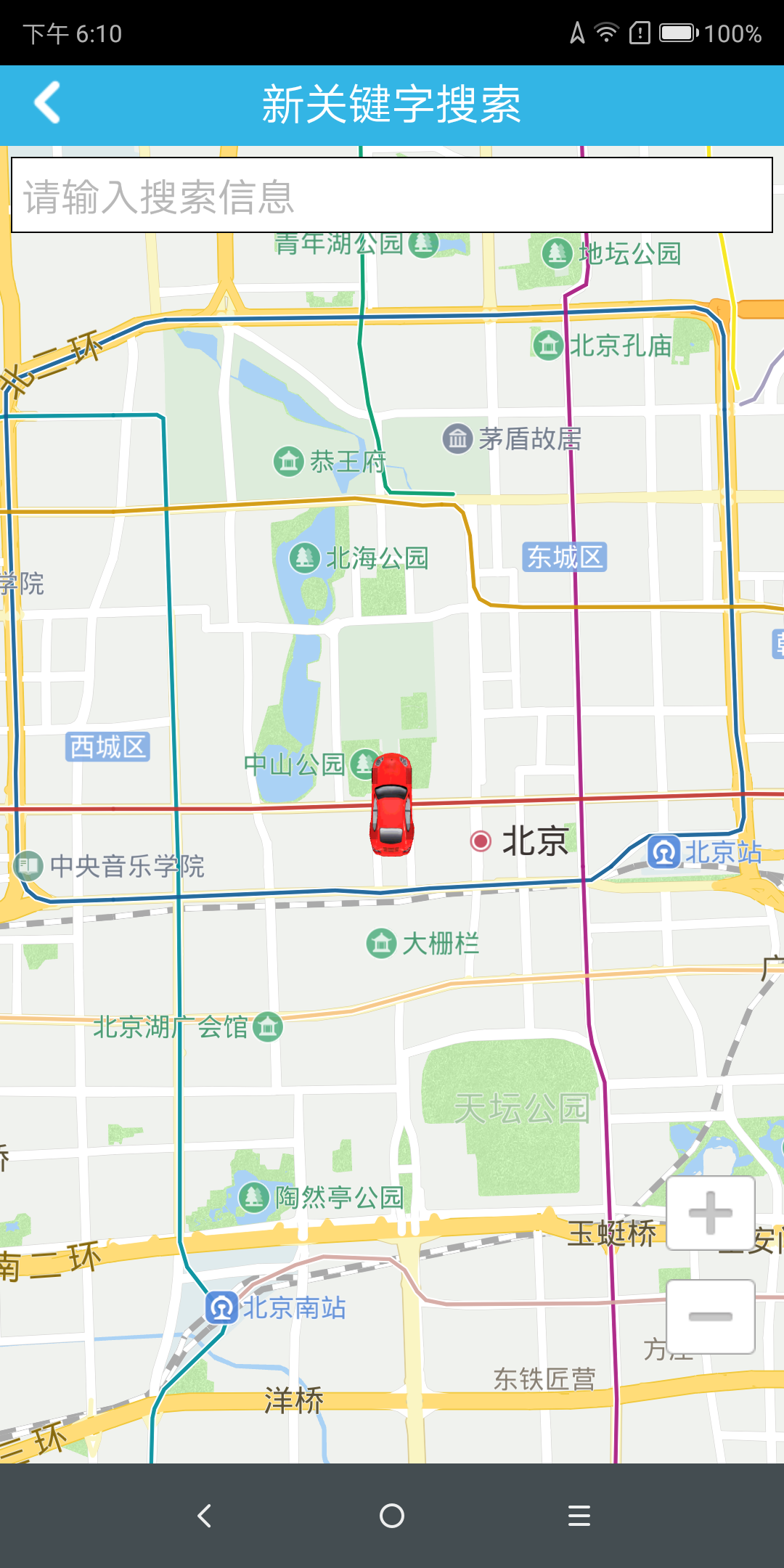
Click the search button to return the result:
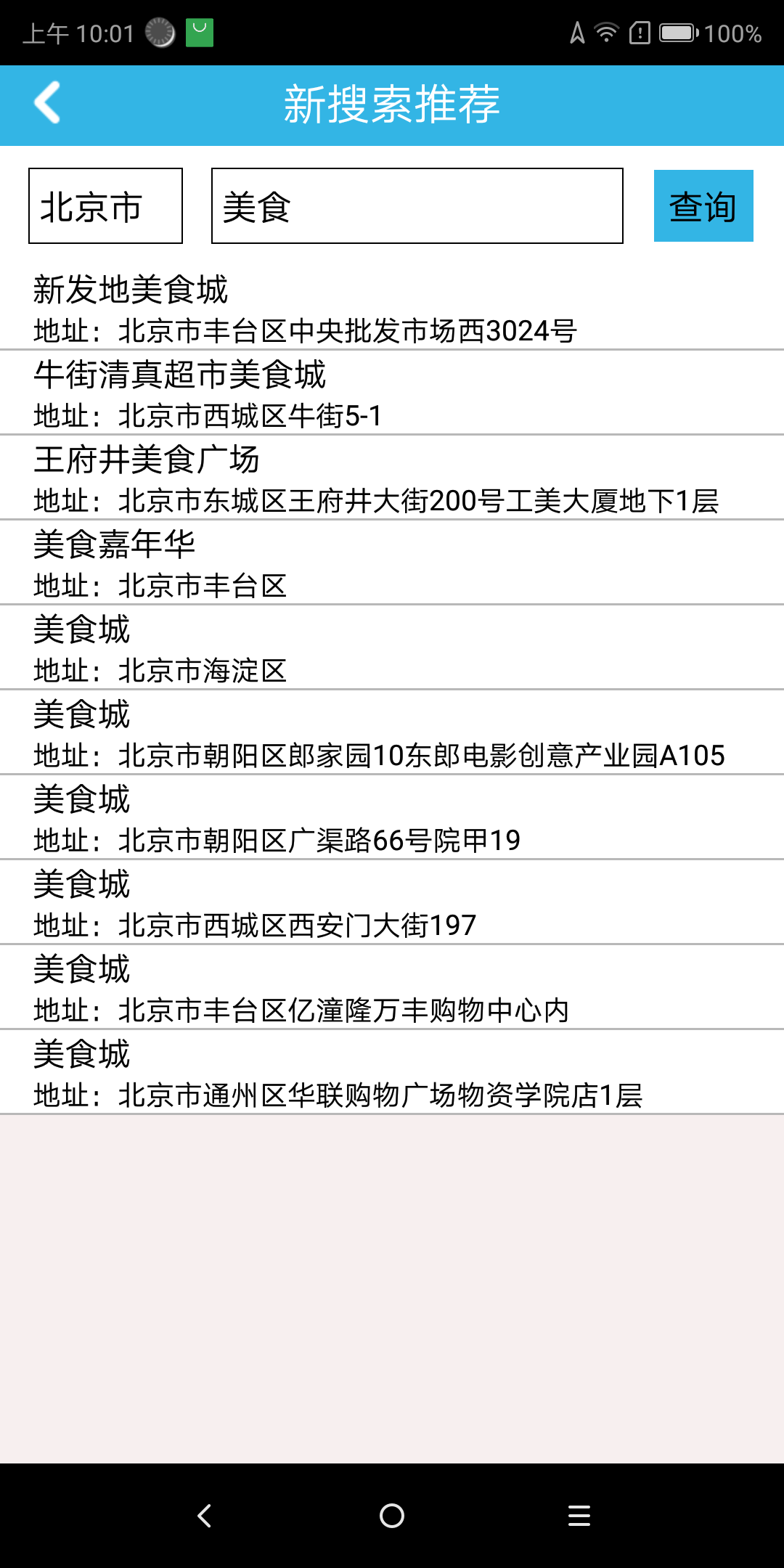