EV Routing
1. Import header files
#import <NavinfoKit/NavinfoKit.h>
2. Configure APIKEY
Refer to Project Configuration Instructions.
3. Define NavinfoRouteSearch
Define the master road object NavinfoRouteSearch and inherit the search protocol <NavinfoRouteQueryDelegate>.
4. Construct NavinfoRouteSearch
Construct the main search object NavinfoRouteSearch and set the proxy.
NavinfoRouteSearch * routeSearch = [[NavinfoRouteSearch alloc] init]; routeSearch.delegate = self;
5. Set the starting route calculation parameters
where orig (starting point coordinates) vias (path point coordinate set can be empty) dest (end point coordinates) query (calculation type). Capacity (how much charge is charged)
- (id)initEleCarWithorig:(NavinfoLonlat * _Nonnull)orig vias:(NSArray<NavinfoLonlat *> * _Nullable)vias dest:(NavinfoLonlat * _Nonnull)dest soc:(double)soc capacity:(double)capacity
6. Initiating driving calculations
Initiate a driving calculation query by calling the startSearch method of NavinfoSearchRoute.
[search startSearchWith:query];
7. Processing data in callbacks
When the query is successful, it will enter the callback function, and the callback function can get the result of the calculation.
Description:
Get a driving and transfer plan set via response.routes.
- (void)onRouteSearch:(NavinfoRouteSearch * _Nonnull)routeSearch result:(NavinfoRouteResult * _Nullable)result error:(NSError * _Nullable)error { NSLog(@"----> onRouteSearch"); }
8. Processing failed queries
When the retrieval fails, Error returns, and the reason for the failure is obtained by the callback function.
- (void)onRouteSearch:(NavinfoRouteSearch * _Nonnull)routeSearch result:(NavinfoRouteResult * _Nullable)result error:(NSError * _Nullable)error { NSLog(@"----> onRouteSearch"); }
Code example:
- (void)routeSearch:(NavinfoQueryType)type{ NavinfoLonlat * busOrig = [[NavinfoLonlat alloc] initWith:11633930.3 latitude:4001116.0 gbType:NavinfoGbTypeG02]; NavinfoLonlat * busDest = [[NavinfoLonlat alloc] initWith:11645256.2 latitude:3993640.4 gbType:NavinfoGbTypeG02]; NavinfoLonlat * orig = [[NavinfoLonlat alloc] initWith:11634000 latitude:4045000 gbType:NavinfoGbTypeG02]; NavinfoLonlat * dest = [[NavinfoLonlat alloc] initWith:11635000 latitude:4054000 gbType:NavinfoGbTypeG02]; NavinfoLonlat * orig1 = [[NavinfoLonlat alloc] initWith:11645000 latitude:4034000 gbType:NavinfoGbTypeG02]; NavinfoLonlat * dest1 = [[NavinfoLonlat alloc] initWith:11646000 latitude:4035000 gbType:NavinfoGbTypeG02]; NavinfoLonlat * carOrig = [[NavinfoLonlat alloc] initWith:12161561 latitude:3891521 gbType:NavinfoGbTypeG02]; NavinfoLonlat * carDest = [[NavinfoLonlat alloc] initWith:12151443 latitude:3884730 gbType:NavinfoGbTypeG02]; NavinfoRouteQuery * query = nil; switch (type) { case QUERYTYPE_BUS: Query = [[NavinfoRouteQuery alloc] initBusQueryWith:busOrig dest:busDest city:@"BEIJING"]; break; case QUERYTYPE_CAR: query = [[NavinfoRouteQuery alloc] initWithorig:carOrig vias:nil dest:carDest]; break; case QUERYTYPE_ELE_CAR: query = [[NavinfoRouteQuery alloc] initEleCarWithorig:orig vias:nil dest:dest soc:20 capacity:40]; break; case QUERYTYPE_BULK: query = [[NavinfoRouteQuery alloc] initBulkQueryWithorigs:@[orig,orig1] dests:@[dest,dest1]]; break; default: break; } NavinfoRouteSearch * search = [[NavinfoRouteSearch alloc]init]; search.delegate = self; [search startSearchWith:query]; } - (void)onRouteSearch:(NavinfoRouteSearch * _Nonnull)routeSearch response:(NavinfoRouteResult * _Nullable)response error:(NSError * _Nullable)error{ }
Run the program, the effect is as follows:
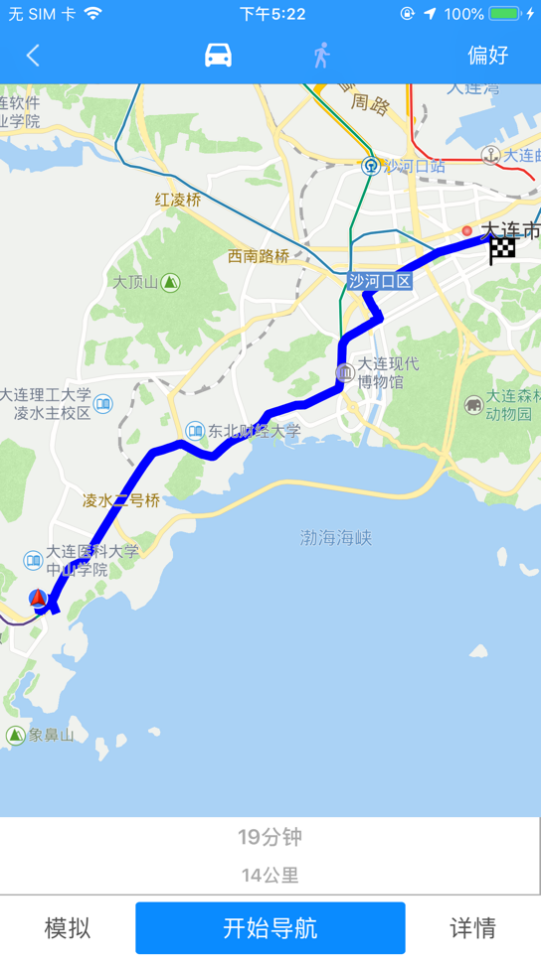
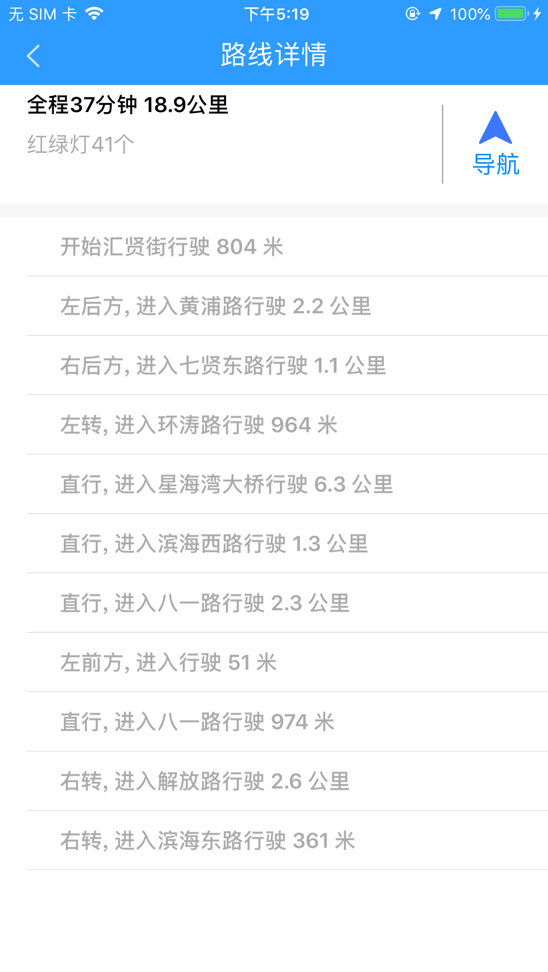
2. Configure APIKEY
Refer to Project Configuration Instructions.
3. Definition NavinfoEleCarReachAreaSearch
Define the tram travel range object NavinfoEleCarReachAreaSearch and inherit the search protocol <NavinfoEleCarReachAreaSearchQueryDelegate>.
4. Construct NavinfoEleCarReachAreaSearch
Construct the main search object NavinfoEleCarReachAreaSearch and set the proxy.
NavinfoEleCarReachAreaSearch * areaSearch = [[NavinfoEleCarReachAreaSearch alloc] init]; areaSearch.delegate = self;
5. Set the parameters for initiating the driving range of the tram
The request parameter class of the piggy query is NavinfoEleCarAreaQuery, and the shortcut constructs the NavinfoEleCarAreaQuery method. The required parameter centerLonlat (self-car position), soc (current car power).
- (id)initWithAreaCenter:(NavinfoLonlat *)centerLonlat soc:(double)soc;
6. Initiate driving range request
Initiate a calculation query by calling the startSearchWith method of NavinfoEleCarReachAreaSearch.
[routeMatch startMatchWith:matchQuery];
7. Processing data in callbacks
When the query is successful, it will enter the callback function, and the callback function can get the result of the calculation.
Description:
Get a collection of points that the tram can reach by response.lonlats.
- (void)onEleCarReachAreaSearch:(NavinfoEleCarReachAreaSearch * _Nonnull)routeSearch response:(NavinfoEleCarReachAreaResult * _Nullable)response error:(NSError * _Nullable)error;
8. Processing failed queries
When the retrieval fails, Error returns, and the reason for the failure is obtained by the callback function.
- (void)onEleCarReachAreaSearch:(NavinfoEleCarReachAreaSearch * _Nonnull)routeSearch response:(NavinfoEleCarReachAreaResult * _Nullable)response error:(NSError * _Nullable)error{ NSLog(@"Error: %@", error); }
Code example:
- (void)reachAreaTest{ NavinfoLonlat * centerLonlat = [[NavinfoLonlat alloc]initWith:11637830 latitude:3989856 gbType:NavinfoGbTypeG02]; NavinfoEleCarAreaQuery * areaQuery = [[NavinfoEleCarAreaQuery alloc]initWithAreaCenter:centerLonlat soc:30]; NavinfoEleCarReachAreaSearch * areaSearch = [[NavinfoEleCarReachAreaSearch alloc]init]; [areaSearch startSearchWith:areaQuery]; } - (void)onEleCarReachAreaSearch:(NavinfoEleCarReachAreaSearch * _Nonnull)routeSearch response:(NavinfoEleCarReachAreaResult * _Nullable)response error:(NSError * _Nullable)error{ NSLog(@"Error: %@", error); }
Run the program, the effect is as shown below:
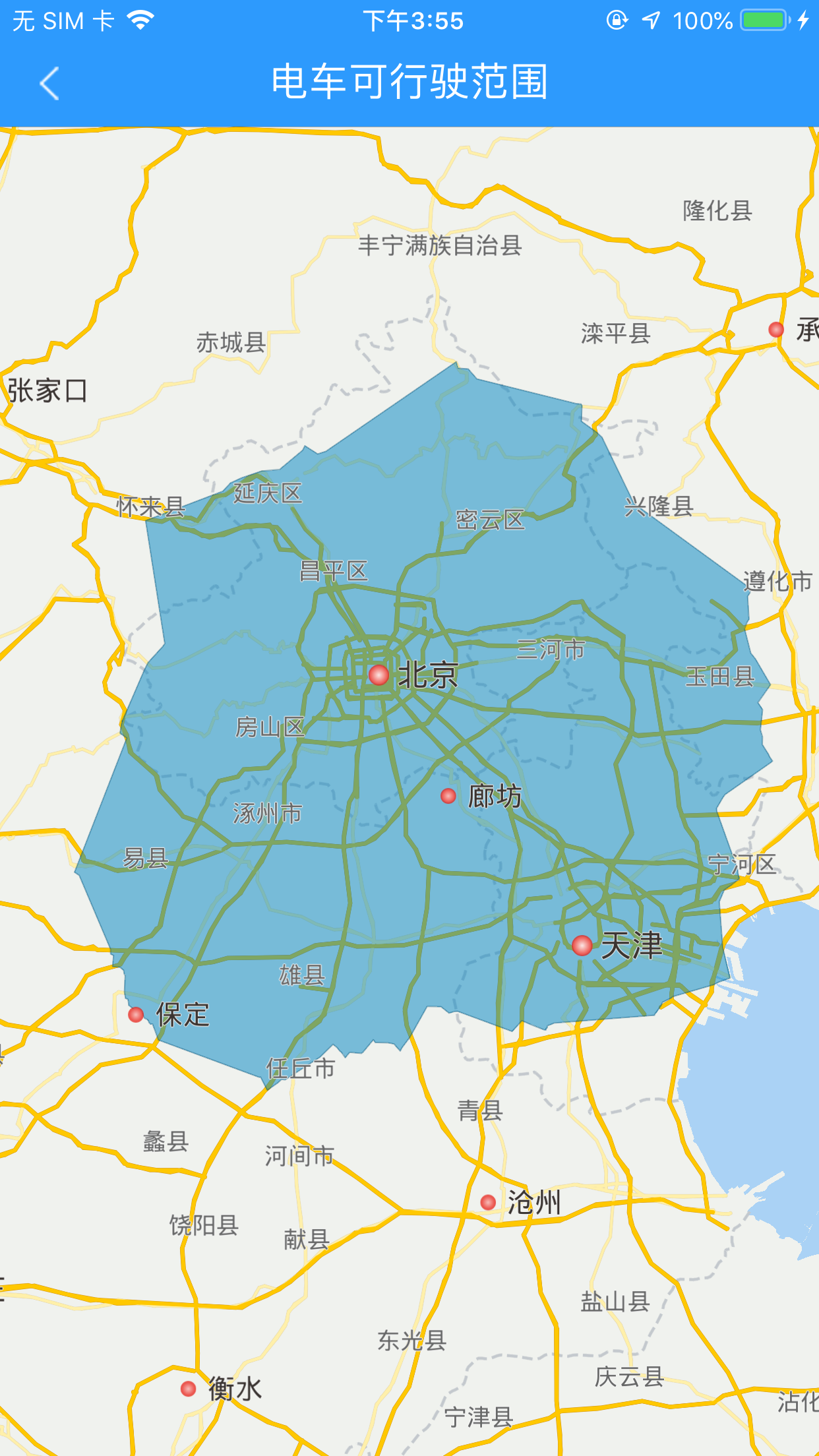