POI Search
Keyword Search
1. Import header files
#import <NavinfoKit/NavinfoKit.h>
2. Configure APIKEY
Refer to Project Configuration Instructions.
3. Define NavinfoPOISearch
Define the main search object NavinfoPOISearch and inherit the search protocol <NavinfoQueryDelegate>.
4. Constructing NavinfoPOISearch
Construct an event search object NavinfoPOISearch and set the proxy.
self.search = [[NavinfoPOISearch alloc] init]; self.search.delegate = self;
5. Set keyword search query parameters
The request parameter class of the keyword search query is NavinfoPOIQuery, and the keyword (keyword), city (city), and category (search type) are mandatory parameters.
The keyword search category is SERVICE_API_SEARCH_TEXT.
Self.query = [[NavinfoPOIQuery alloc] initWithKeyword:@"HOME INN" city:@"BEIJING" lonlats:nil category:SERVICE_API_SEARCH_TEXT];
6. Initiate keyword search query parameters
Initiate a keyword search query by calling the search method of NavinfoPOISearch.
[self.search search:self.query];
7. Processing data in callbacks
When the query is successful, it will go to the POISearch:result:error callback function, through the callback function, you can get the result of the query keyword search.
- (void)POISearch:(NavinfoPOISearch *)poiSearch result:(NavinfoPOISearchResult *)result error:(NSError *)error
Description:
1) Get the total number of results retrieved by result. total.
2) Get the identifier of the filtering sorting configuration information by result.
3) Get a list of province distribution results by result. provinces. See NavinfoProvince for details.
4) Get a list of POI messages via result pois. Refer to NavinfoPOI for details.
5) Obtain administrative area switching information through result. For more information, please refer to NavinfoDistrict
8. Processing a failed query When the retrieval fails, Error returns, and the reason for the failure is obtained by the callback function.
Run the program, the effect is as shown below:
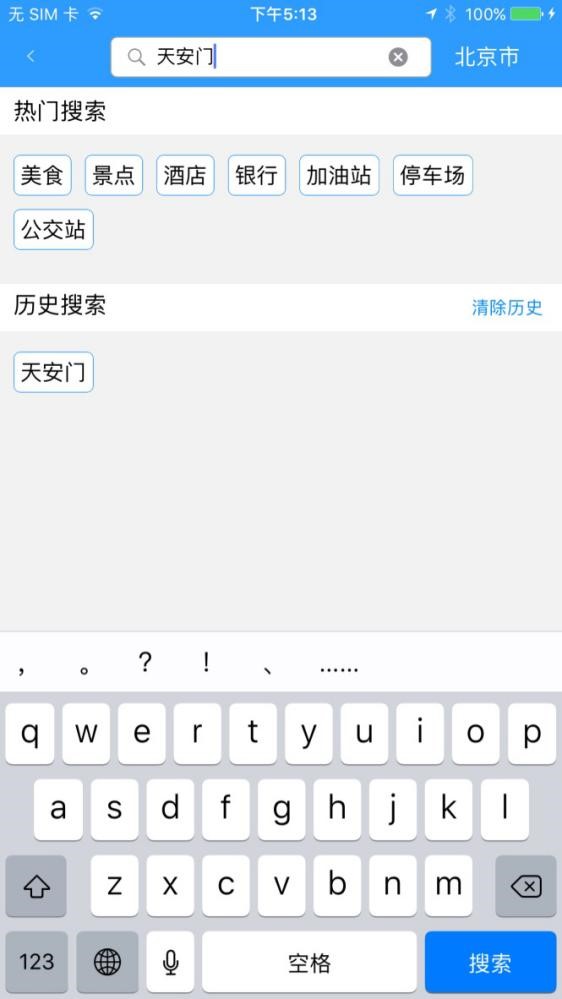
Circular Search
Refer to the keyword search in detail, the different settings are as follows:
5. Set circular search query parameters
The request parameter class of the circular search query is NavinfoPOIQuery, keyword (keyword), city (city), lonlat (current position coordinate), category (search type) are mandatory parameters.
The circle search category is SERVICE_API_SEARCH_AROUND.
Self.query = [[NavinfoPOIQuery alloc] initWithKeyword:@"FOOD" city:@"BEIJING" lonlats:[NavinfoLonlat toLonlatsWithLonlatStrings:@"116.311050,39.980570"] category:SERVICE_API_SEARCH_AROUND];
Rectangle Search
Refer to the keyword search in detail, the different settings are as follows:
5. Set rectangular search query parameters
The request parameter class of the rectangle search query is NavinfoPOIQuery, keyword (keyword), city (city), lonlats (rectangular coordinate string), category (search type) are mandatory parameters.
Rectangle search category is SERVICE_API_SEARCH_POLYGON.
NSString *lonlatString = @"116.311050,39.980570;116.310880,39.945280;116.335770,39.926260;116.359210,39.923100"; Self.query = [[NavinfoPOIQuery alloc] initWithKeyword:@"FOOD" city:@"BEIJING" lonlats:[NavinfoLonlat toLonlatsWithLonlatStrings:lonlatString] category:SERVICE_API_SEARCH_AROUND];
Search Along the Way
Refer to the keyword search in detail, the different settings are as follows:
5. Set search query parameters along the way
The request parameter class of the search query along the way is NavinfoPOIQuery, keyword (keyword), city (city), lonlats (rectangular coordinate string), category (search type) are required parameters.
Search for category along the route to SERVICE_API_SEARCH_LINE.
NSString *lonlatString = @"116.311050,39.980570;116.310880,39.945280;116.335770,39.926260;116.359210,39.923100"; Self.query = [[NavinfoPOIQuery alloc] initWithKeyword:@"FOOD" city:@"BEIJING" lonlats:[NavinfoLonlat toLonlatsWithLonlatStrings:lonlatString] category: SERVICE_API_SEARCH_LINE];
Details Query
1. Import header files
#import <NavinfoKit/NavinfoKit.h>
2. Configure APIKEY
Refer to Project Configuration Instructions.
3. Define NavinfoDetailsSearch
Define the main search object NavinfoDetailsSearch and inherit the search protocol <NavinfoQueryDelegate>.
4. Construct NavinfoDetailsSearch
Construct an event search object NavinfoDetailsSearch and set the proxy.
self.detailSearch= [[NavinfoDetailsSearch alloc] init]; self.detailSearch.delegate = self;
5. Set location details to retrieve query parameters
The request parameter class for location details retrieval is NavinfoDetailsQuery, poiId (POI unique id) and category (search type) are mandatory parameters.
poiId is the information of the poi carried in the search results, so you can only search first and then view the location details.
Location details retrieve category as SERVICE_API_SEARCH_DETAIL.
self.detailQuery = [[NavinfoDetailsQuery alloc] init]; self.detailQuery.category = SERVICE_API_SEARCH_DETAIL;
6. Initiate location details search query
Initiate a location details retrieval query by calling the getLocationDetails method of NavinfoDetailsSearch.
self.detailSearch getLocationDetails:[result.pois objectAtIndex:0].poiId query:self.detailQuery];
7. Processing data in callbacks
When the query is successful, it will go to the locationDetails:result:error callback function, through the callback function, you can get the results of the query location details search.
- (void)POISearch:(NavinfoPOISearch *)poiSearch result:(NavinfoPOISearchResult *)result error:(NSError *)error
Description:
1) Get the total number of results retrieved by result. total.
2) Get the identifier of the filtering sorting configuration information by result.
3) Get a list of province distribution results by result. provinces. See NavinfoProvince for details.
4) Get a list of POI messages via result pois. Refer to NavinfoPOI for details.
5) Obtain administrative area switching information through result. See NavinfoDistrict for details.
8. Processing failed queries
When the retrieval fails, Error returns, and the reason for the failure is obtained by the callback function.
Run the program, the effect is as shown below:
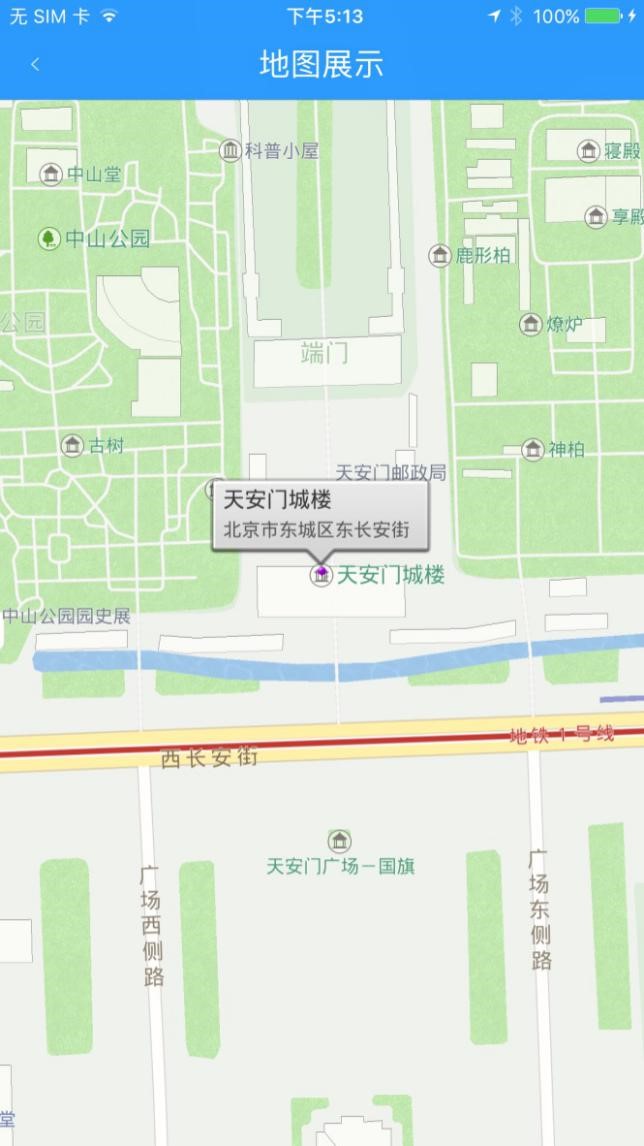
Partner Information Query
1. Import header files
2. Configure APIKEY
#import <NavinfoKit/NavinfoKit.h>
Refer to Project Configuration Instructions.
3. Define NavinfoCooperationDetailsSearch
Define the main search object NavinfoCooperationDetailsSearch and inherit the search protocol <NavinfoCooperationQueryDelegate>.
4. Construct NavinfoCooperationDetailsSearch
Construct an event search object NavinfoCooperationDetailsSearch and set the proxy.
5. Set partner location details search parameters
self.cooperationDetailSearch= [[NavinfoCooperationDetailsSearch alloc] init]; self.cooperationDetailSearch.delegate = self;
The request parameter class retrieved by the partner location details is NavinfoCooperationDetailsQuery, poiId (POI unique id) and poiName (POI unique name) are mandatory parameters.
poiId, poiName is the information of the poi carried in the search results, so you can only search first, then view the location details.
6. Initiate Partner Location Details Search
self.cooperationDetailQuery = [[NavinfoCooperationDetailsQuery alloc] init];
Initiate a partner location details retrieval query by calling the getCooperationLocationDetails method of NavinfoCooperationDetailsSearch.
7. Processing data in callbacks
[self.cooperationDetailSearch getCooperationLocationDetails:[result.pois objectAtIndex:0].extend.cooperationId cooperationName:[result.pois objectAtIndex:0].extend.cooperationName query:self.cooperationDetailQuery];
When the query is successful, it will go to the locationCooperationDetails:result:error callback function. Through the callback function, you can get the result of the query partner location details search.
Description:
- (void)locationCooperationDetails:(NavinfoCooperationDetailsSearch *)poiSearch result:(NavinfoCooperationInfomationResult *)result error:(NSError *)error
1) Obtain the partner location details unique identifier by result. cooperationId.
2) Get the partner location details name via result. cooperationName.
3) Get the partner location details coordinates via result. lonlat.
4) Obtain the partner location details address via result. address.
5) Get the partner location details city by result. city.
6) Get the partner location details call via result. phone.
7) Get the partner location details business hours via result.officeTime.
8) Get the partner location details category by result. type, hotel: hotel, cater: food, scenery: attractions parking: parking lot.
9) Get the partner location details I through result. rinfo.
10) Get the cover map of the partner location details by result. rImageUrl.
11) Get the partner location details facility via result. facility Only the hotel and food have data to return.
12) Get the partner location details recommendation information through result. recommend type=scenery, return to the ticket purchase notice (only the attraction has data to return).
13) Get up to 5 photo points of the partner's location details via result. rImageUrls.
14) Get the partner location details extension information by result. extend. This field is displayed only when exinfo = 2, different poi types, and the displayed content is different.
8. Processing failed queries
When the retrieval fails, Error returns, and the reason for the failure is obtained by the callback function.